PHP Exercises: Create a new string without the first and last character of a given string of any length
Write a PHP program to create a new string without the first and last character of a given string of any length.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that extracts the middle portion of a string, excluding the first and last characters
function test($s1)
{
// Check if the string has less than 2 characters and return an empty string if true
return strlen($s1) < 2 ? "" : substr($s1, 1, strlen($s1) - 2);
}
// Test the 'test' function with different strings and display the results
echo test("Hello")."\n";
echo test("JS")."\n";
echo test(" ")."\n";
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes one parameter, $s1, representing the input string.
- Length Check:
- The function checks if the length of the string $s1 is less than 2:
- If true (the string has 0 or 1 character), it returns an empty string "".
- If false (the string has 2 or more characters), it proceeds to the next step.
- Substring Extraction:
- If the string length is sufficient, it uses the substr function to extract the middle portion of the string:
- substr($s1, 1, strlen($s1) - 2):
- This extracts a substring starting from the second character (index 1) up to the second-to-last character.
- The length of the extracted substring is calculated as strlen($s1) - 2, effectively removing the first and last characters from the original string.
Output:
ell
Flowchart:
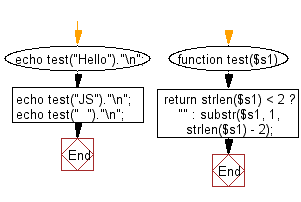
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to move the last two characters to the start of a given string of length at least two.
Next: Write a PHP program to create a new string using the two middle characters of a given string of even length (at least 2).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics