PHP Exercises: Move the first two characters to the end of a given string of length at least two
67. Move First Two Characters to End
Write a PHP program to move the first two characters to the end of a given string of length at least two.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that rearranges the characters of a string
function test($s1)
{
// Use substr to extract substrings and rearrange them
return substr($s1, 2, strlen($s1) - 2) . substr($s1, 0, 2);
}
// Test the 'test' function with different strings and display the results
echo test("Hello")."\n";
echo test("JS");
?>
Explanation:
- Function Definition:
- The function test is defined to take one string parameter, $s1.
- Character Rearrangement:
- Inside the function, two substr calls are used to rearrange the characters of the input string $s1:
- substr($s1, 2, strlen($s1) - 2) extracts a substring starting from the third character to the end of the string.
- substr($s1, 0, 2) extracts the first two characters of the string.
- Concatenation:
- The function returns the concatenation of the two extracted substrings, effectively moving the first two characters to the end of the string.
Output:
lloHe JS
Flowchart:
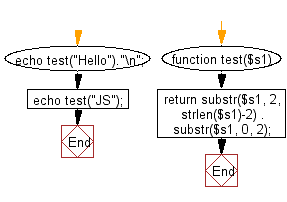
For more Practice: Solve these Related Problems:
- Write a PHP script to reposition the first two characters of an input string to its end while preserving the rest of the string.
- Write a PHP function to rotate a string by moving its initial two characters to the tail and return the new formation.
- Write a PHP program to implement a custom string rotation algorithm that shifts the first two letters to the end.
- Write a PHP script to simulate cyclic string shifting by transferring the first two characters to the back of the string.
Go to:
PREV : Remove First Character from Both and Concat.
NEXT : Move Last Two Characters to Start.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.