PHP Exercises: Concat two given string of length atleast 1, after removing their first character
Write a PHP program to concat two given string of length atleast 1, after removing their first character.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that concatenates substrings excluding the first character
function test($s1, $s2)
{
// Use substr to extract substrings excluding the first character and concatenate them
return substr($s1, 1, strlen($s1) - 1) . substr($s2, 1, strlen($s2) - 1);
}
// Test the 'test' function with different pairs of strings and display the results
echo test("Hello", "Hi")."\n";
echo test("JS", "Python")."\n";
?>
Explanation:
- Function Definition:
- The function test is defined to take two string parameters, $s1 and $s2.
- Substring Extraction:
- Inside the function, substr is used to extract substrings from both $s1 and $s2, excluding their first characters:
- substr($s1, 1, strlen($s1) - 1) extracts all characters from $s1 starting from the second character.
- substr($s2, 1, strlen($s2) - 1) does the same for $s2.
- Concatenation:
- The function returns the concatenated result of these substrings from $s1 and $s2.
Output:
elloi Sython
Flowchart:
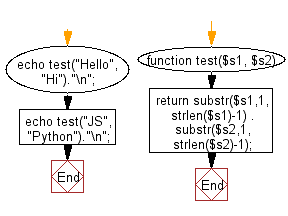
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string from two given string one is shorter and another is longer. The format of the new string will be long string + short string + long string.
Next: Write a PHP program to move the first two characters to the end of a given string of length at least two.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics