PHP Exercises: Create a new string without the first and last character of a given string of length atleast two
PHP Basic Algorithm: Exercise-64 with Solution
Write a PHP program to create a new string without the first and last character of a given string of length atleast two.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that manipulates the substring of s1
function test($s1)
{
// Use substr to exclude the first character, then another substr to exclude the last two characters
return substr(substr($s1, 1, strlen($s1) - 1), 0, strlen($s1) - 2);
}
// Test the 'test' function with different input strings and display the results
echo test("Hello")."\n";
echo test("Hi")."\n";
echo test("Python")."\n";
?>
Sample Output:
ell ytho
Flowchart:
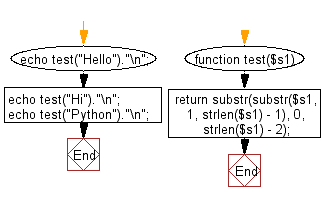
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string of the first half of a given string of even length.
Next: Write a PHP program to create a new string from two given string one is shorter and another is longer. The format of the new string will be long string + short string + long string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics