PHP Exercises: Create a new string using first two characters of a given string
Write a PHP program to create a new string using first two characters of a given string. If the string length is less than 2 then return the original string.
Sample Solution:
PHP Code :
Explanation:
- Function Definition:
- The function test is defined to take one parameter, $s1, which is expected to be a string.
- Length Check:
- The function first checks if the length of $s1 is less than 2 using strlen($s1) < 2.
- Return Logic:
- If Length < 2:
- If the length is less than 2, it returns $s1 as is (meaning the entire string, whether it's empty or a single character).
- If Length ≥ 2:
- If the length is 2 or more, it uses substr($s1, 0, 2) to extract the first two characters of the string and returns them.
Output:
He Hi H
Flowchart:
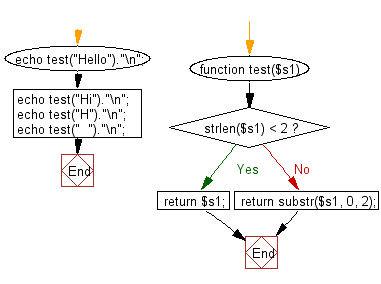
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string using three copies of the last two character of a given string of length atleast two.
Next: Write a PHP program to create a new string of the first half of a given string of even length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics