PHP Exercises: Insert a given string into middle of the another given string of length 4
PHP Basic Algorithm: Exercise-60 with Solution
Write a PHP program to insert a given string into middle of the another given string of length 4.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that inserts a word into a string at a specific position
function test($s1, $word)
{
// Use substr to concatenate the first two characters of s1, followed by the 'word', and then the rest of s1
return substr($s1, 0, 2) . $word . substr($s1, 2);
}
// Test the 'test' function with different input strings and display the results
echo test("[[]]", "Hello")."\n";
echo test("(())", "Hi");
?>
Explanation:
- Function Purpose:
- The test function takes two parameters, $s1 (a string) and $word (a string to insert), and inserts $word into $s1 at the third position (after the first two characters).
- Insertion Logic:
- The function uses substr to split $s1 into two parts:
- The first two characters of $s1 (substr($s1, 0, 2)).
- The rest of $s1 from the third character onward (substr($s1, 2)).
- It then concatenates the parts: the first two characters of $s1, followed by $word, followed by the rest of $s1.
Output:
[[Hello]] ((Hi))
Flowchart:
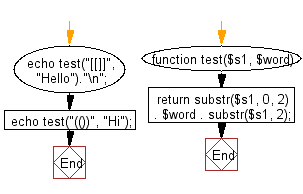
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string using two given strings s1, s2, the format of the new string will be s1s2s2s1.
Next: Write a PHP program to create a new string using three copies of the last two character of a given string of length atleast two.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics