PHP Exercises: Remove the character in a given position of a given string
Write a PHP program to remove the character in a given position of a given string. The given position will be in the range 0..string length -1 inclusive.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters, $s and $n
function test($s, $n)
{
// Use the substr function to concatenate the substring of $s from index 0 to $n-1
// with the substring of $s from index $n+1 to the end of the string
return substr($s, 0, $n) . substr($s, $n + 1, strlen($s) - $n);
}
// Call the test function with arguments "Python" and 1, then echo the result
echo test("Python", 1) . "\n";
// Call the test function with arguments "Python" and 0, then echo the result
echo test("Python", 0) . "\n";
// Call the test function with arguments "Python" and 4, then echo the result
echo test("Python", 4) . "\n";
?>
Explanation:
- Function Definition:
- The function test takes two parameters: a string $s and an integer $n.
- Substrings and Concatenation:
- The function uses substr to:
- Extract the part of $s from the start (index 0) up to (but not including) the character at index $n.
- Concatenate this with the part of $s from index $n + 1 to the end, effectively skipping the character at index $n.
- Function Purpose:
- This function removes the character at index $n from the string $s.
- Function Calls and Output:
- First Call: test("Python", 1)
- Removes the character at index 1 ("y"), resulting in "Pthon".
- Second Call: test("Python", 0)
- Removes the character at index 0 ("P"), resulting in "ython".
- Third Call: test("Python", 4)
- Removes the character at index 4 ("o"), resulting in " Pythn".
Output:
Pthon ython Pythn
Visual Presentation:
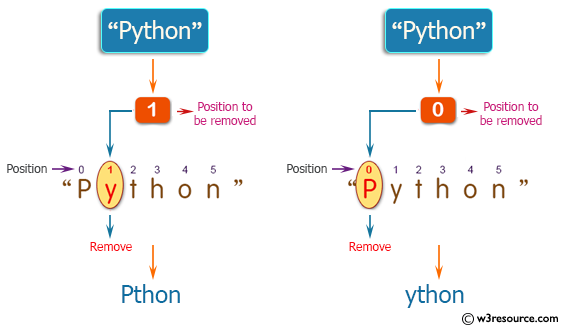
Flowchart:
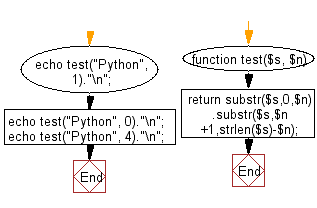
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
Next: Write a PHP program to exchange the first and last characters in a given string and return the new string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics