PHP Exercises: Create a new string where 'if' is added to the front of a given string
Write a PHP program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $s
function test($s)
{
// Check if the length of $s is greater than or equal to 2 AND if the substring of $s from index 0 to 1 is equal to "if"
if (strlen($s) >= 2 && substr($s, 0, 2) == "if")
{
// If true, return $s as it is
return $s;
}
// If false, prepend "if " to $s and return the result
return "if " . $s;
}
// Call the test function with argument "if else" and echo the result
echo test("if else") . "\n";
// Call the test function with argument "else" and echo the result
echo test("else") . "\n";
// Call the test function with argument "if" and echo the result
echo test("if") . "\n";
?>
Explanation:
- Function Definition:
- The function test is defined with a single parameter $s.
- Condition Check:
- The function checks two conditions:
- The length of $s is at least 2 characters (strlen($s) >= 2).
- The first two characters of $s are "if" (substr($s, 0, 2) == "if").
- If both conditions are true, it returns $s unchanged.
- Return Value:
- If either condition is false, the function prepends "if " to $s and returns the modified string.
- Function Calls and Output:
- First Call: echo test("if else")
- The string starts with "if", so it returns "if else" unchanged.
- Second Call: echo test("else")
- The string does not start with "if", so it returns "if else".
- Third Call: echo test("if")
- The string starts with "if", so it returns "if" unchanged.
Output:
if else if else if
Flowchart:
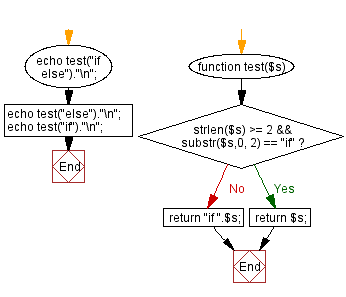
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a given integer and return true if it is within 10 of 100 or 200.
Next: Write a PHP program to remove the character in a given position of a given string. The given position will be in the range 0..string length -1 inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics