PHP Exercises: Check if it is possible to add two integers to get the third integer from three given integers
46. Two Integers Sum to Third Check
Write a PHP program to check if it is possible to add two integers to get the third integer from three given integers.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if any two numbers add up to the third number
function test($x, $y, $z)
{
// Check if $x is equal to the sum of $y and $z
// OR if $y is equal to the sum of $x and $z
// OR if $z is equal to the sum of $x and $y
return $x == $y + $z || $y == $x + $z || $z == $x + $y;
}
// Test the function with different sets of numbers
var_dump(test(1, 2, 3))."\n";
var_dump(test(4, 5, 6))."\n";
var_dump(test(-1, 1, 0))."\n";
?>
Explanation:
- Function Definition:
- The function test checks if any two numbers from the inputs $x, $y, and $z add up to the third number.
- Conditions Checked:
- Condition 1: Checks if $x is equal to the sum of $y and $z.
- Condition 2: Checks if $y is equal to the sum of $x and $z.
- Condition 3: Checks if $z is equal to the sum of $x and $y.
- If any of these conditions are true, the function returns true; otherwise, it returns false.
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
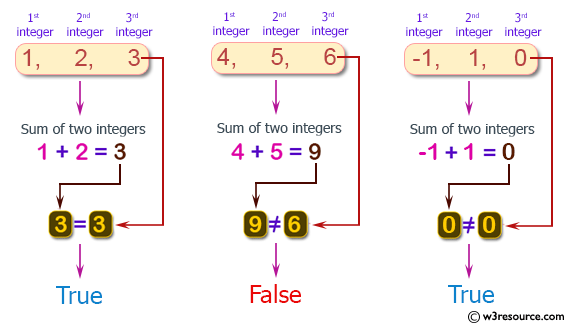
Flowchart:
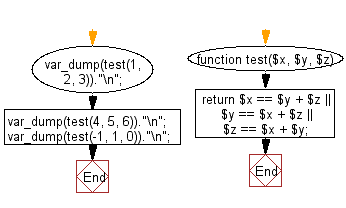
For more Practice: Solve these Related Problems:
- Write a PHP script to check if any two out of three given integers add up exactly to the third integer.
- Write a PHP function to iterate through combinations of three numbers and return true if a valid sum equation is found.
- Write a PHP program to use nested loops to verify if any two numbers in a triplet can be summed to equal the third.
- Write a PHP script to implement a combinatorial check for three inputs to determine if the addition of one pair results in the remaining number.
Go to:
PREV : FizzBuzz String Start with F and/or End with B.
NEXT : Increasing Order Check for x,y,z.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.