PHP Exercises: Check if a given non-negative given number is a multiple of 3 or 7, but not both
Write a PHP program to check if a given non-negative given number is a multiple of 3 or 7, but not both.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a number satisfies an exclusive OR (^) condition involving modulo operations
function test($n)
{
// Check if the remainder of $n divided by 3 is 0 XOR the remainder of $n divided by 7 is 0
return ($n % 3 == 0) ^ ($n % 7 == 0);
}
// Test the function with different values
var_dump(test(3));
var_dump(test(7));
var_dump(test(21));
?>
Explanation:
- Function Definition:
- The test function checks if a given number $n satisfies a specific condition using the exclusive OR (XOR) operator ^ with modulo operations.
- Condition Checked:
- The function evaluates whether:
- $n is divisible by 3 (i.e., $n % 3 == 0), XOR
- $n is divisible by 7 (i.e., $n % 7 == 0).
- The XOR operator returns true only if one of these conditions is true but not both.
- If either condition is met exclusively, the function returns true; otherwise, it returns false.
Output:
int(1) int(1) int(0)
Visual Presentation:
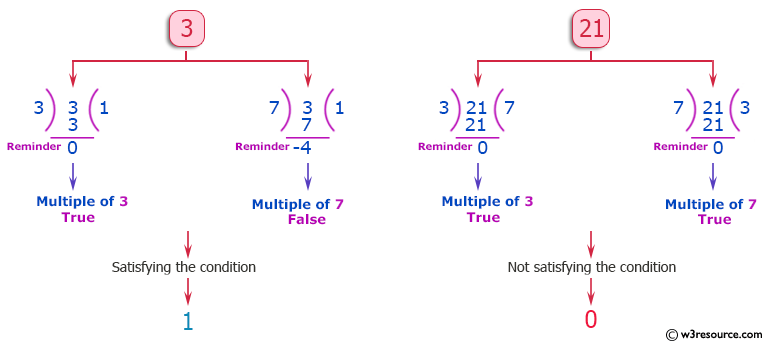
Flowchart:
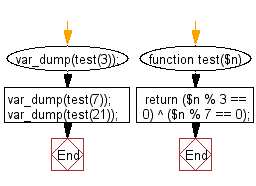
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to test if a given non-negative number is a multiple of 13 or it is one more than a multiple of 13.
Next: Write a PHP program to check if a given number is within 2 of a multiple of 10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics