PHP Exercises: Accept two integers and return true if either one is 5 or their sum or difference is 5
40. Check for 5, Sum or Difference Equals 5
Write a PHP program that accept two integers and return true if either one is 5 or their sum or difference is 5.
Sample Solution:
PHP Code :
<?php
// Define a function that checks conditions related to the numbers
function test($x, $y)
{
// Check if $x is 5 or $y is 5 or the sum of $x and $y is 5
// or the absolute difference between $x and $y is 5
return $x == 5 || $y == 5 || $x + $y == 5 || abs($x - $y) == 5;
}
// Test the function with different pairs of numbers
var_dump(test(5, 4));
var_dump(test(4, 3));
var_dump(test(1, 4));
?>
Explanation:
- Function Definition:
- The test function takes two numbers, $x and $y, as parameters and checks several conditions.
- Conditions Checked:
- It checks if:
- $x is equal to 5.
- $y is equal to 5.
- The sum of $x and $y is 5.
- The absolute difference between $x and $y is 5.
- If any of these conditions are true, the function returns true; otherwise, it returns false.
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
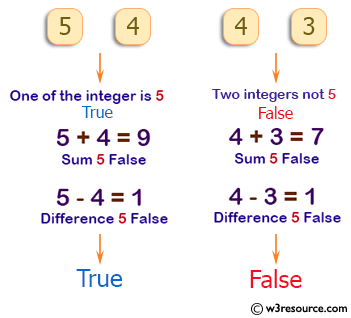
Flowchart:
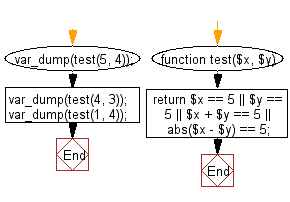
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if either of two integers equals 5, or if their sum or absolute difference equals 5.
- Write a PHP function to verify whether two numbers satisfy the condition of having 5 as a value or result from arithmetic operations.
- Write a PHP program to check combinations of two numbers and return a boolean if any arithmetic relation equals 5.
- Write a PHP script to test two integers for direct equality to 5 or for sum/difference equality to 5 using nested conditions.
Go to:
PREV : Sum with Conditional Range Return 30.
NEXT : Multiple of 13 or One More Than Multiple.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.