PHP Exercises: Check a given integer and return true if it is within 10 of 100 or 200
4. Within 10 of 100 or 200
Write a PHP program to check a given integer and return true if it is within 10 of 100 or 200.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $x
function test($x)
{
// Use the absolute value function (abs) to check if the absolute difference between $x and 100 is less than or equal to 10
// OR if the absolute difference between $x and 200 is less than or equal to 10
if (abs($x - 100) <= 10 || abs($x - 200) <= 10)
// If true, return true
return true;
// If false, return false
return false;
}
// Use var_dump to print the result of calling test with argument 103
var_dump(test(103));
// Use var_dump to print the result of calling test with argument 90
var_dump(test(90));
// Use var_dump to print the result of calling test with argument 89
var_dump(test(89));
?>
Explanation:
- Function Definition:
- The function test is defined with a single parameter $x.
- Condition Check:
- The function checks if:
- The absolute difference between $x and 100 is less than or equal to 10, or
- The absolute difference between $x and 200 is less than or equal to 10.
- It uses the abs function to calculate the absolute difference, ensuring it works correctly with both positive and negative values.
- If either condition is met, the function returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: var_dump(test(103));
- abs(103 - 100) is 3, which is within 10, so it returns true.
- Second Call: var_dump(test(90));
- abs(90 - 100) is 10, which is within 10, so it returns true.
- Third Call: var_dump(test(89));
- abs(89 - 100) is 11, which is more than 10, so it returns false.
Output:
bool(true) bool(true) bool(false)
Flowchart:
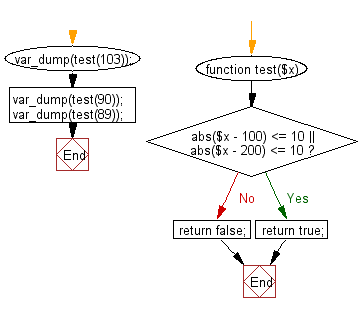
For more Practice: Solve these Related Problems:
- Write a PHP function to check if a number is within 10 units of 100 or 200, using absolute value comparisons.
- Write a PHP script to return true if an integer is within a tolerance of 10 from either 100 or 200, with input validation for negative numbers.
- Write a PHP program to test if a number lies within a 10-unit range of 100 or 200 by combining conditional operators.
- Write a PHP script to perform the proximity check to 100 and 200 without using built-in abs() and using custom math logic.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Next: Write a PHP program to create a new string where 'if' is added to the front of a given string. If the string already begins with 'if', return the string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.