PHP Exercises: Compute the sum of the two given integers
39. Sum with Conditional Range Return 30
Write a PHP program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
Sample Solution:
PHP Code :
<?php
// Define a function that checks the sum of two numbers
function test($a, $b)
{
// Check if the sum of $a and $b is between 10 and 20 (inclusive)
// If true, return 30; otherwise, return the actual sum
return $a + $b >= 10 && $a + $b <= 20 ? 30 : $a + $b;
}
// Test the function with different pairs of numbers
echo test(12, 17)."\n";
echo test(2, 17)."\n";
echo test(22, 17)."\n";
echo test(20, 0)."\n";
?>
Explanation:
- Function Definition:
- The test function takes two numbers, $a and $b, as inputs and checks the sum against a specific range.
- Sum Calculation and Range Check:
- It calculates the sum of $a and $b.
- If the sum is between 10 and 20 (inclusive), it returns 30.
- If the sum is outside this range, it returns the actual sum of $a and $b.
Output:
29 30 39 30
Visual Presentation:
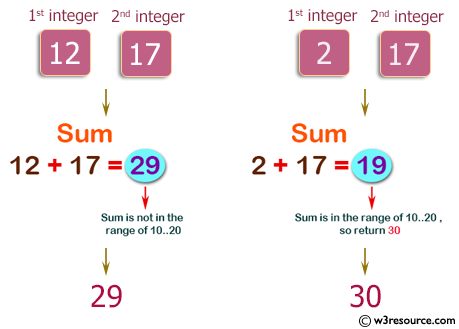
Flowchart:
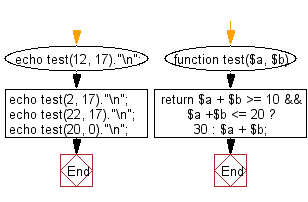
For more Practice: Solve these Related Problems:
- Write a PHP script to sum two integers and return 30 if the result falls within the inclusive range of 10 to 20; otherwise, return the original sum.
- Write a PHP function to calculate the sum of two numbers and conditionally adjust the result when the sum falls within a specific range.
- Write a PHP program to add two integers and apply a rule that outputs a fixed value of 30 when the sum is within a given boundary.
- Write a PHP script to use logical checks to override the computed sum with 30 if it meets the range criteria of 10 to 20 inclusive.
Go to:
PREV : Detect Triple Consecutive Occurrence.
NEXT : Check for 5, Sum or Difference Equals 5.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.