PHP Exercises: Check if a triple is presents in an array of integers or not
Write a PHP program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
Sample Solution:
PHP Code :
<?php
// Define a function that checks for consecutive identical elements in an array
function test($nums)
{
// Calculate the length of the array
$arra_len = sizeof($nums) - 1;
// Initialize a variable to store the current element
$n = 0;
// Iterate through the array up to the second-to-last element
for ($i = 0; $i < $arra_len; $i++)
{
// Retrieve the current element
$n = $nums[$i];
// Check if the current, next, and next-next elements are identical
if ($n == $nums[$i + 1] && $n == $nums[$i + 2])
{
// Return true if the condition is met
return true;
}
}
// Return false if the condition is not met
return false;
}
// Test the function with different arrays
var_dump(test(array(1, 1, 2, 2, 1)));
var_dump(test(array(1, 1, 2, 1, 2, 3)));
var_dump(test(array(1, 1, 1, 2, 2, 2, 1)));
?>
Explanation:
- Function Definition:
- The test function accepts an array of numbers, $nums, and checks if it contains three consecutive identical elements.
- Array Length Calculation:
- $arra_len is set to the length of $nums minus 1, allowing the loop to safely access the last set of three consecutive elements.
- Loop through the Array:
- A for loop iterates through $nums up to the second-to-last element, ensuring that $nums[$i + 2] is a valid index.
- Retrieve Current Element:
- The current element $n is set to $nums[$i].
- Check for Three Consecutive Identical Elements:
- The if statement checks if the current element $n is equal to the next two elements ($nums[$i + 1] and $nums[$i + 2]).
- If this condition is met, the function immediately returns true, indicating three consecutive identical elements were found.
- Return false if No Consecutive Identicals Found:
- If the loop completes without finding three consecutive identical elements, the function returns false.
Output:
bool(false) bool(false) bool(true)
Visual Presentation:
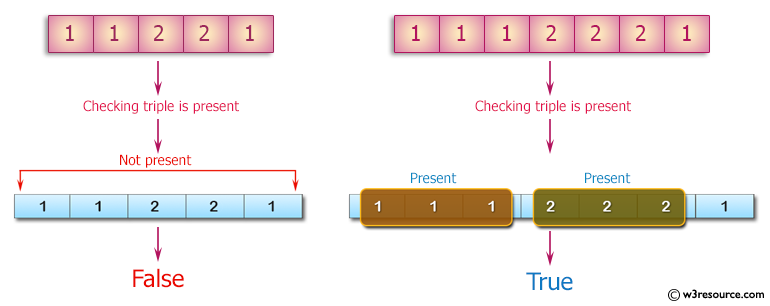
Flowchart:
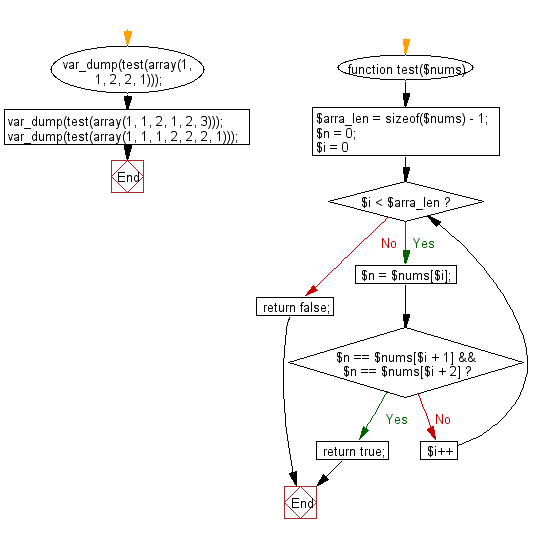
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
Next: Write a PHP program to compute the sum of the two given integers. If the sum is in the range 10..20 inclusive return 30.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics