PHP Exercises: Count the number of two 5's are next to each other in an array of integers
37. Count Adjacent 5's with 6 Variation
Write a PHP program to count the number of two 5's are next to each other in an array of integers. Also count the situation where the second 5 is actually a 6.
Sample Solution:
PHP Code :
<?php
// Define a function that counts the occurrences of specific patterns in an array
function test($numbers)
{
// Initialize a counter variable
$ctr = 0;
// Iterate through the array up to the second-to-last element
for ($i = 0; $i < sizeof($numbers) - 1; $i++)
{
// Check if the current and next elements form a specific pattern
if (($numbers[$i] == 5) && ($numbers[$i + 1] == 5) || ($numbers[$i + 1] == 6))
{
// Increment the counter if the pattern is found
$ctr++;
}
}
// Return the final count
return $ctr;
}
// Test the function with different arrays
echo test([5, 5, 2])."\n";
echo test([5, 5, 2, 5, 5])."\n";
echo test([5, 6, 2, 9])."\n";
?>
Explanation:
- Function Definition:
- The test function takes an array of numbers, $numbers, as input and counts occurrences of specific patterns.
- Initialize Counter:
- A variable $ctr is initialized to 0 to keep track of the pattern matches found.
- Loop through the Array:
- A for loop iterates through $numbers from the start to the second-to-last element, using sizeof($numbers) - 1 as the limit.
- Pattern Check:
- The if condition checks if the current element ($numbers[$i]) is 5 and the next element ($numbers[$i + 1]) is either:
- 5 (two consecutive 5’s) or
- 6 (5 followed by 6).
- If either pattern is found, $ctr is incremented by 1.
- Return the Result:
- After the loop completes, the function returns the final count of pattern occurrences stored in $ctr.
Output:
1 2 1
Visual Presentation:
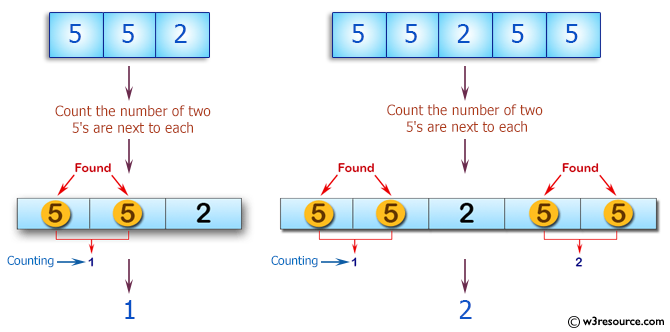
Flowchart:
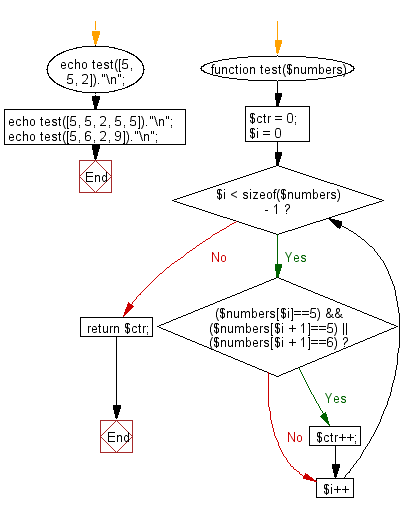
For more Practice: Solve these Related Problems:
- Write a PHP script to count pairs of adjacent 5's in an array, also counting a 5 followed by 6 as a valid pair.
- Write a PHP function to iterate through an array and tally adjacent occurrences of the number 5, allowing for a trailing 6.
- Write a PHP program to scan an integer array and detect consecutive number patterns where 5 can be followed by either 5 or 6.
- Write a PHP script to check every pair of adjacent numbers in an array and count them if they meet the criteria for 5 and alternate 6.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string of the characters at indexes 0,1,4,5,8,9 ... from a given string.
Next: Write a PHP program to check if a triple is presents in an array of integers or not. If a value appears three times in a row in an array it is called a triple.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.