PHP Exercises: Compare two given strings and return the number of the positions where they contain the same length 2 substring
35. Compare Strings for Matching 2-Character Substrings
Write a PHP program to compare two given strings and return the number of the positions where they contain the same length 2 substring.
Sample Solution:
PHP Code :
<?php
// Define a function that counts the number of common two-character substrings between two strings
function test($s1, $s2)
{
// Initialize a counter variable
$ctr = 0;
// Iterate through substrings of the first string
for ($i = 0; $i < strlen($s1)-1; $i++)
{
// Extract a two-character substring from the first string
$firstString = substr($s1, $i, 2);
// Iterate through substrings of the second string
for ($j = 0; $j < strlen($s2)-1; $j++)
{
// Extract a two-character substring from the second string
$secondString = substr($s2, $j, 2);
// Check if the two substrings are equal, and increment the counter if they are
if ($firstString == $secondString)
$ctr++;
}
}
// Return the final count of common two-character substrings
return $ctr;
}
// Test the function with different pairs of strings
echo (test("abcdefgh", "abijsklm"))."\n";
echo (test("abcde", "osuefrcd"))."\n";
echo (test("pqrstuvwx", "pqkdiewx"))."\n";
?>
Explanation:
- Function Definition:
- The test function takes two strings, $s1 and $s2, and counts the number of common two-character substrings between them.
- Counter Initialization:
- A variable $ctr is initialized to keep track of the count of matching two-character substrings.
- Iterate through $s1:
- The outer for loop iterates through each possible two-character substring in $s1.
- For each index i, substr($s1, $i, 2) extracts a two-character substring from $s1, stored in $firstString.
- Iterate through $s2:
- The inner for loop iterates through each possible two-character substring in $s2.
- For each index j, substr($s2, $j, 2) extracts a two-character substring from $s2, stored in $secondString.
- Compare Substrings:
- If $firstString is equal to $secondString, the counter $ctr is incremented.
- Return Result:
- After all comparisons, the function returns $ctr, representing the number of common two-character substrings between $s1 and $s2.
Output:
1 1 2
Flowchart:
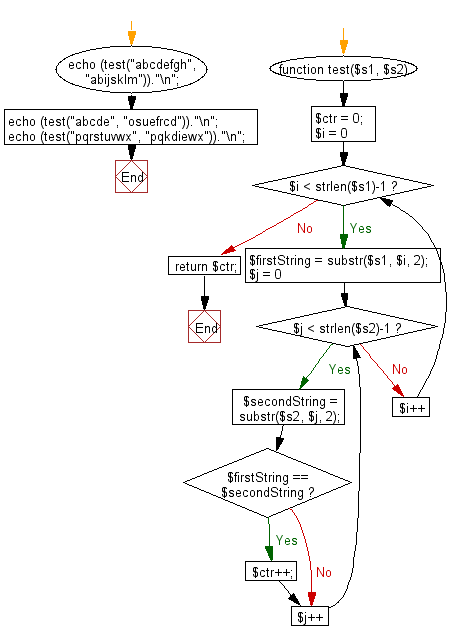
For more Practice: Solve these Related Problems:
- Write a PHP script to compare two strings and count how many positions have the same two-character substring.
- Write a PHP function to slide through two strings in parallel and tally the number of identical two-character pairs.
- Write a PHP program to iterate over indices of two strings and compare pairs of characters at the same positions.
- Write a PHP script to compare substrings of length two from two input strings and return the count of matching positions.
Go to:
PREV : Check for Sequence 1,2,3 in Array.
NEXT : New String from Specific Indexes.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.