PHP Exercises: Check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere
PHP Basic Algorithm: Exercise-34 with Solution
Write a PHP program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if the sequence 1, 2, 3 appears in an array
function test($nums)
{
// Iterate through the array to find the sequence
for ($i = 0; $i < sizeof($nums)-2; $i++)
{
// Check if the current, next, and next-next elements form the sequence 1, 2, 3
if ($nums[$i] == 1 && $nums[$i + 1] == 2 && $nums[$i + 2] == 3)
return true;
}
// If the sequence is not found, return false
return false;
}
// Test the function with different arrays
var_dump(test(array(1,1,2,3,1)));
var_dump(test(array(1,1,2,4,1)));
var_dump(test(array(1,1,2,1,2,3)));
?>
Sample Output:
bool(true) bool(false) bool(true)
Visual Presentation:
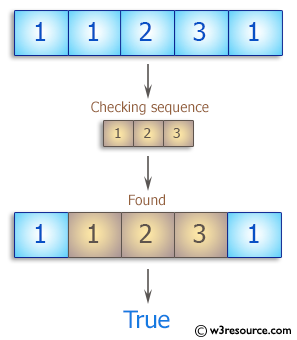
Flowchart:
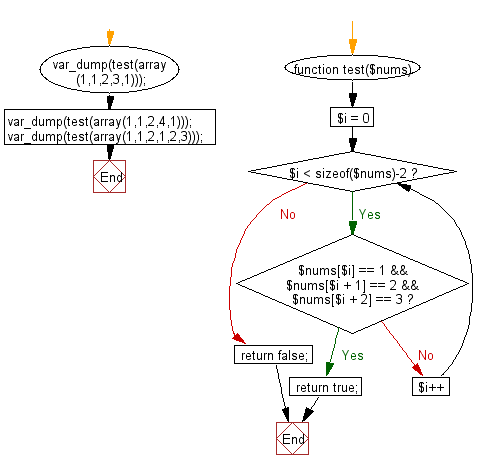
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if one of the first 4 elements in an array of integers is equal to a given element.
Next: Write a PHP program to compare two given strings and return the number of the positions where they contain the same length 2 substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics