PHP Exercises: Check if one of the first 4 elements in an array of integers is equal to a given element
PHP Basic Algorithm: Exercise-33 with Solution
Write a PHP program to check if one of the first 4 elements in an array of integers is equal to a given element.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a given number is present in an array of numbers
function test($nums, $n)
{
// Use the sizeof function to check the size of the array and determine the range for in_array
return sizeof($nums) < 4 ? in_array($n, $nums) : in_array($n, array_slice($nums, 0, 4));
}
// Test the function with different arrays and numbers
var_dump(test(array(1,2,9,3), 3));
var_dump(test(array(1,2,3,4,5,6), 2));
var_dump(test(array(1,2,2,3), 9));
?>
Sample Output:
bool(true) bool(true) bool(false)
Visual Presentation:
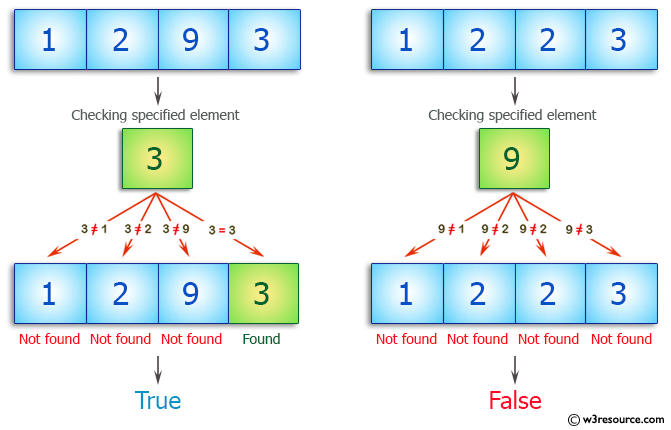
Flowchart:
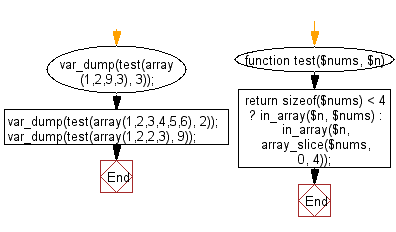
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a specified number is present in a given array of integers.
Next: Write a PHP program to check whether the sequence of numbers 1, 2, 3 appears in a given array of integers somewhere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics