PHP Exercises: Create a string like "aababcabcd" from a given string "abcd"
Write a PHP program to create a string like "aababcabcd" from a given string "abcd".
Sample Solution:
PHP Code :
<?php
// Define a function that generates a string by concatenating substrings from the input string
function test($s)
{
// Initialize an empty string to store the result
$result = "";
// Iterate through the string
for ($i = 0; $i < strlen($s); $i++) {
// Append substrings from the beginning up to the current index
$result .= substr($s, 0, $i + 1);
}
// Return the final result
return $result;
}
// Test the function with different input strings
echo test("abcd")."\n";
echo test("abc")."\n";
echo test("a")."\n";
?>
Explanation:
- Function Definition:
- The test function takes a single parameter, $s, which is a string. It builds and returns a new string by concatenating progressively longer substrings from $s.
- Initialize Result Variable:
- An empty string $result is initialized to store the concatenated substrings.
- Iterate Through String:
- A for loop iterates through each character of the string $s, from index 0 to strlen($s) - 1.
- Append Substrings:
- For each index $i, a substring from the beginning of $s up to the character at position $i is appended to $result.
- substr($s, 0, $i + 1) extracts this substring.
- Return Final Result:
- After the loop, $result contains the cumulative substrings and is returned.
Output:
aababcabcd aababc a
Flowchart:
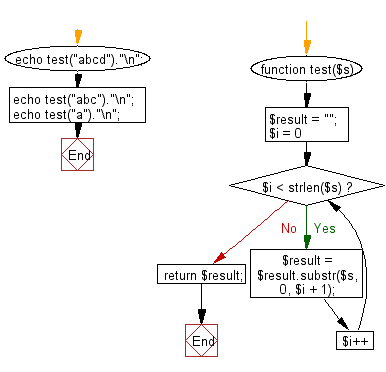
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string made of every other character starting with the first from a given string.
Next: Write a PHP program to count a substring of length 2 appears in a given string and also as the last 2 characters of the string. Do not count the end substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics