PHP Exercises: Check two given integers, and return true if one of them is 30 or if their sum is 30
PHP Basic Algorithm: Exercise-3 with Solution
Write a PHP program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters, $x and $y
function test($x, $y)
{
// Use the logical OR operator to check if $x is equal to 30, $y is equal to 30, or the sum of $x and $y is equal to 30
return ($x == 30) || ($y == 30) || ($x + $y == 30);
}
// Use var_dump to print the result of calling test with arguments 30 and 0
var_dump(test(30, 0));
// Use var_dump to print the result of calling test with arguments 25 and 5
var_dump(test(25, 5));
// Use var_dump to print the result of calling test with arguments 20 and 30
var_dump(test(20, 30));
// Use var_dump to print the result of calling test with arguments 20 and 25
var_dump(test(20, 25));
?>
Explanation:
- Function Definition:
- The function test is defined with two parameters, $x and $y.
- Logical Check:
- The function checks if:
- $x is equal to 30, or
- $y is equal to 30, or
- The sum of $x and $y is equal to 30.
- It uses the logical OR (||) operator, meaning that if any one of these conditions is true, the function will return true. Otherwise, it returns false.
- Function Calls and Output:
- First Call: var_dump(test(30, 0));
- Since $x is 30, it returns true.
- Second Call: var_dump(test(25, 5));
- The sum of $x and $y is 30 (25 + 5), so it returns true.
- Third Call: var_dump(test(20, 30));
- Since $y is 30, it returns true.
- Fourth Call: var_dump(test(20, 25));
- None of the conditions are met, so it returns false.
Output:
bool(true) bool(true) bool(true) bool(false)
Visual Presentation:
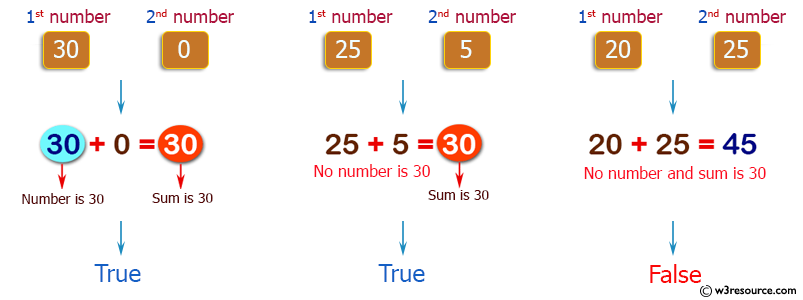
Flowchart:
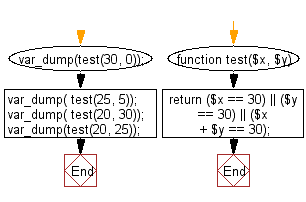
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of the two given integer values. If the two values are the same, then returns triple their sum.
Next: Write a PHP program to check a given integer and return true if it is within 10 of 100 or 200.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics