PHP Exercises: Check if the first appearance of "a" in a given string is immediately followed by another "a"
28. First "a" Followed Immediately by Another "a"
Write a PHP program to check if the first appearance of "a" in a given string is immediately followed by another "a".
Sample Solution:
PHP Code :
<?php
// Define a function that checks for the presence of at least two consecutive 'a's in a string
function test($s)
{
// Initialize a counter for 'a' occurrences
$counter = 0;
// Iterate through the string
for ($i = 0; $i < strlen($s) - 1; $i++) {
// Check for 'a' and increment the counter
if (substr($s, $i, 1) == 'a') {
$counter++;
}
// Check for 'aa' and if counter is less than 2, return true
if ((substr($s, $i, 2) == 'aa') && $counter < 2) {
return true;
}
}
// If no condition met, return false
return false;
}
// Test the function with different input strings
var_dump(test("caabb"));
var_dump(test("babaaba"));
var_dump(test("aaaaa"));
?>
Explanation:
- Function Definition:
- The test function takes one parameter, $s, which is a string. It checks if there are two consecutive 'a' characters ("aa") with only one or no 'a' characters before it.
- Initialize Counter:
- A variable $counter is initialized to 0 to count occurrences of individual 'a' characters.
- Loop Through String:
- A for loop iterates through each character of the string up to the second-to-last character (strlen($s) - 1).
- Count Single 'a' Characters:
- Within the loop, if the character at the current position is 'a', $counter is incremented by 1.
- Check for Consecutive "aa":
- If two consecutive characters ("aa") are found, the function checks if $counter is less than 2.
- If true, it returns true, meaning at least one "aa" was found with fewer than 2 previous 'a' characters.
- Return False if No "aa" Matches Found:
- If the loop completes without finding "aa" that meets the condition, the function returns false.
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
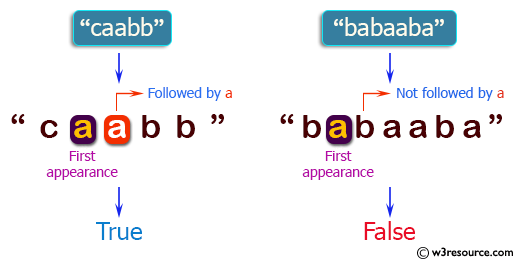
Flowchart:
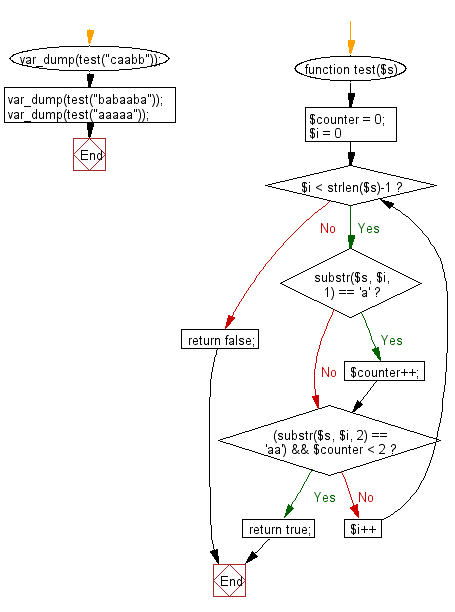
For more Practice: Solve these Related Problems:
- Write a PHP script to check if the first occurrence of the letter "a" in a string is directly followed by another "a".
- Write a PHP function to find the first "a" in a string and verify if the next character is also "a" using substring indexing.
- Write a PHP program to parse a string and determine if an "a" is immediately repeated after its first appearance.
- Write a PHP script to use regex to match the pattern "aa" at the beginning portion of the string and output a boolean result.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to count the string "aa" in a given string and assume "aaa" contains two "aa".
Next: Write a PHP program to create a new string made of every other character starting with the first from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.