PHP Exercises: Create a new string which is n copies of the the first 3 characters of a given string
26. n Copies of First 3 Characters
Write a PHP program to create a new string which is n (non-negative integer) copies of the the first 3 characters of a given string. If the length of the given string is less than 3 then return n copies of the string.
Sample Solution:
PHP Code :
<?php
// Define a function that repeats the front part of a string $n times
function test($s, $n)
{
// Initialize an empty string to store the result
$result = "";
// Define the number of characters from the front of the string to repeat
$frontOfString = 3;
// Adjust $frontOfString if it exceeds the length of the input string
if ($frontOfString > strlen($s)) {
$frontOfString = strlen($s);
}
// Extract the front part of the string
$front = substr($s, 0, $frontOfString);
// Concatenate the front part to the result $n times
for ($i = 0; $i < $n; $i++) {
$result = $result . $front;
}
// Return the final result
return $result;
}
// Test the function with different inputs
echo test("Python", 2) . "\n";
echo test("Python", 3) . "\n";
echo test("JS", 3) . "\n";
?>
Explanation:
- Function Definition:
- The test function takes two parameters: $s (a string to work with) and $n (the number of times to repeat the front part of the string).
- Initialize Result Variable:
- An empty string variable $result is initialized to accumulate the repeated front part of the string.
- Define Front Part Length:
- A variable $frontOfString is set to 3, indicating the number of characters to take from the beginning of the string.
- Adjust Front Part Length If Needed:
- If the length of $s is shorter than 3, $frontOfString is adjusted to the length of $s to avoid out-of-bounds errors.
- Extract Front Part of the String:
- Using substr, the front portion (either the first three characters or the full string if it’s shorter than three characters) is extracted into $front.
- Repeat the Front Part:
- A for loop runs $n times, concatenating $front to $result in each iteration.
- Return Result:
- The function returns the accumulated $result.
Output:
PytPyt PytPytPyt JSJSJS
Visual Presentation:
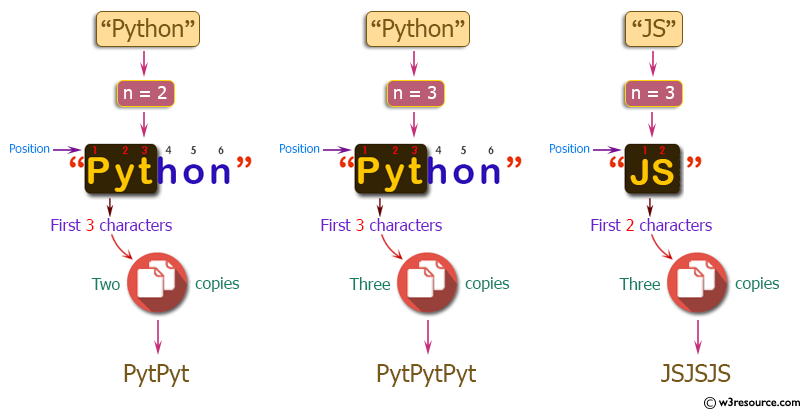
Flowchart:
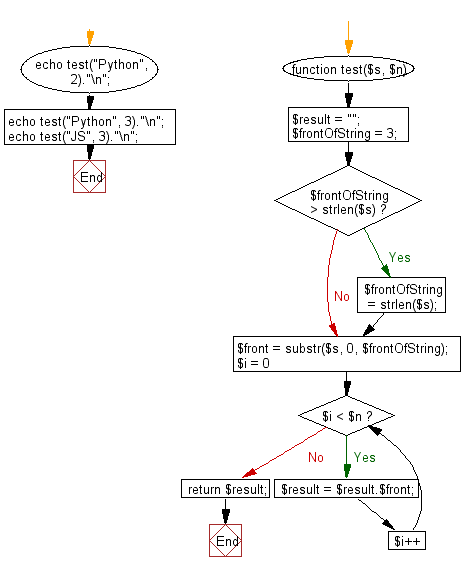
For more Practice: Solve these Related Problems:
- Write a PHP script to create a new string that consists of n copies of the first three characters of an input string, with a fallback for strings shorter than three characters.
- Write a PHP function to extract the first three characters and repeat them n times, using substring functions and loop constructs.
- Write a PHP program to conditionally duplicate either the full string or its leading trio based on string length, multiplied by n.
- Write a PHP script to take a string and an integer n, then build a new string with the appropriate repeated segment, ensuring type safety.
Go to:
PREV : n Copies of a Given String.
NEXT : Count "aa" Substring Occurrences.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.