PHP Exercises: Convert the last 3 characters of a given string in upper case
24. Uppercase Last 3 Characters of String
Write a PHP program to convert the last 3 characters of a given string in upper case. If the length of the string has less than 3 then uppercase all the characters.
Sample Solution:
PHP Code :
<?php
// Define a function that modifies a string based on its length
function test($s)
{
// Check if the length of the string is less than 3
return strlen($s) < 3 ? strtoupper($s) : substr($s, 0, strlen($s) - 3) . strtoupper(substr($s, strlen($s) - 3));
}
// Test the function with different strings
echo test("Python") . "\n";
echo test("Javascript") . "\n";
echo test("js") . "\n";
echo test("PHP") . "\n";
?>
Explanation:
- Function Definition:
- The test function takes a single parameter $s, a string, and modifies it based on its length.
- String Modification Logic:
- If the length of $s is less than 3 characters:
- The entire string is converted to uppercase using strtoupper.
- If the length of $s is 3 characters or more:
- The last 3 characters are converted to uppercase.
- The modified string is created by concatenating the unaltered part of $s (up to strlen($s) - 3) with the uppercase version of the last 3 characters.
Output:
PytHON JavascrIPT JS PHP
Visual Presentation:
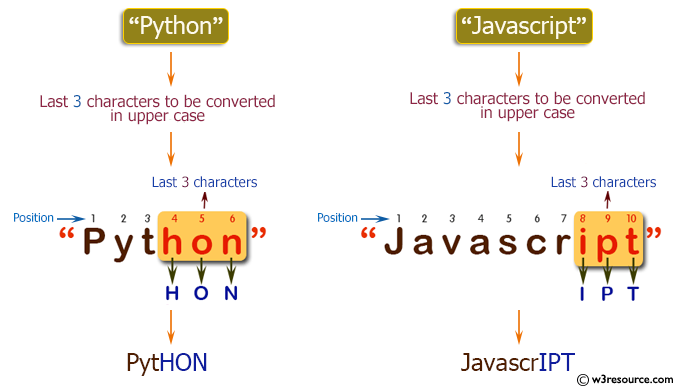
Flowchart:
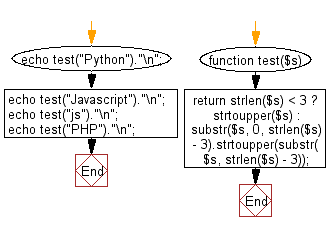
For more Practice: Solve these Related Problems:
- Write a PHP script to convert the final three characters of a string to uppercase, or the entire string if its length is less than three.
- Write a PHP function to check the string length and selectively transform the last three characters to uppercase using substr and strtoupper.
- Write a PHP program to split the string into two parts and apply an uppercase transformation to the latter part conditionally.
- Write a PHP script to simulate the conversion of the last three characters by iterating over the string from the end and updating them accordingly.
Go to:
PREV : Same Last Digit Check.
NEXT : n Copies of a Given String
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.