PHP Exercises: Check if two given non-negative integers have the same last digit
Write a PHP program to check if two given non-negative integers have the same last digit.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if the last digits of two numbers have the same absolute value
function test($x, $y)
{
// Check if the absolute value of the last digit of both numbers is the same
return abs($x % 10) == abs($y % 10);
}
// Test the function with different number pairs
var_dump(test(123, 456));
var_dump(test(12, 512));
var_dump(test(7, 87));
var_dump(test(12, 45));
?>
Explanation:
- Function Definition:
- The test function takes two integer parameters $x and $y and checks if their last digits have the same absolute value.
- Last Digit Comparison:
- The last digit of each number is obtained using the modulus operator (% 10), which provides the remainder when divided by 10.
- The abs function ensures that the comparison is based on the absolute value of the last digits (to handle negative numbers).
- It then checks if the last digits are the same and returns true if they are; otherwise, it returns false.
Output:
bool(false) bool(true) bool(true) bool(false)
Visual Presentation:
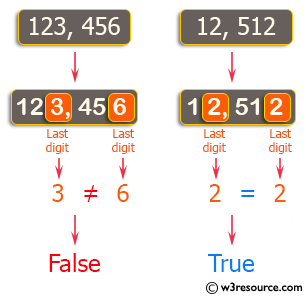
Flowchart:
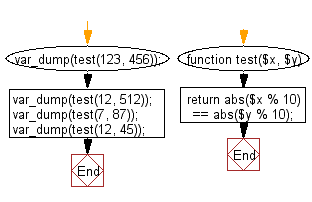
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if a given string contains between 2 and 4 'z' character.
Next: Write a PHP program to convert the last 3 characters of a given string in upper case. If the length of the string has less than 3 then uppercase all the characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics