PHP Exercises: Check if a given string contains between 2 and 4 'z' character
22. Count 'z' Characters Between 2 and 4
Write a PHP program to check if a given string contains between 2 and 4 'z' character.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if a string contains the character 'z' 2 to 3 times
function test($s)
{
// Initialize a counter variable
$ctr = 0;
// Loop through each character in the string
for ($i = 0; $i < strlen($s); $i++)
{
// Check if the current character is 'z' and increment the counter if true
if (substr($s, $i, 1) == 'z')
{
$ctr++;
}
}
// Return true if the counter is greater than 1 and less than 4, indicating 'z' occurs 2 to 3 times
return $ctr > 1 && $ctr < 4;
}
// Test the function with different strings
var_dump(test("frizz"));
var_dump(test("zane"));
var_dump(test("Zazz"));
var_dump(test("false"));
?>
Explanation:
- Function Definition:
- The test function takes a string parameter $s and checks if it contains the character 'z' exactly 2 or 3 times.
- Counter Initialization:
- A counter variable $ctr is initialized to 0. This counter will track the occurrences of 'z'.
- Loop through Each Character:
- A for loop iterates over each character in the string $s.
- Condition Check:
- For each character, it checks if it is 'z'.
- If it is, the counter $ctr is incremented by 1.
- Return Condition:
- After the loop, it returns true if the counter value $ctr is between 2 and 3, inclusive (indicating 2 or 3 occurrences of 'z'). Otherwise, it returns false.
Output:
bool(true) bool(false) bool(true) bool(false)
Visual Presentation:
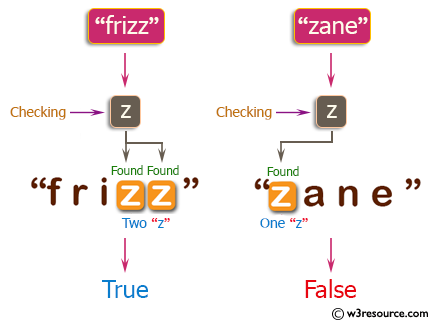
Flowchart:
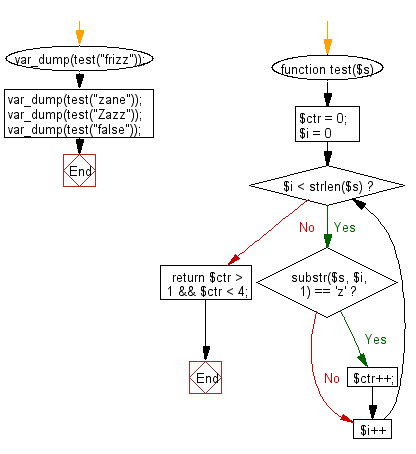
For more Practice: Solve these Related Problems:
- Write a PHP script to count the occurrence of the letter 'z' in a string and check if the count is between 2 and 4 inclusive.
- Write a PHP function to analyze a string for 'z' characters and return true if their frequency meets the specified range.
- Write a PHP program to use regex to count 'z's in an input string and validate the count against set constraints.
- Write a PHP script to scan a string and conditionally output a boolean based on whether the total 'z' count is within the desired limits.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find the larger value from two positive integer values that is in the range 20..30 inclusive, or return 0 if neither is in that range.
Next: Write a PHP program to check if two given non-negative integers have the same last digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.