PHP Exercises: Check if a string 'yt' appears at index 1 in a given string
PHP Basic Algorithm: Exercise-17 with Solution
Write a PHP program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if the substring starting from the second position (index 1) is "yt"
// If true, the function returns a modified string excluding the character at index 1
// If false, the function returns the original string
function test($s)
{
return substr($s, 1, 2) == "yt" ? substr($s, 0, 1).substr($s, 3, strlen($s)-2) : $s;
}
// Test the function with various strings
echo test("Python")."\n";
echo test("ytade")."\n";
echo test("jsues")."\n";
?>
Sample Output:
Phon ytade jsues
Visual Presentation:
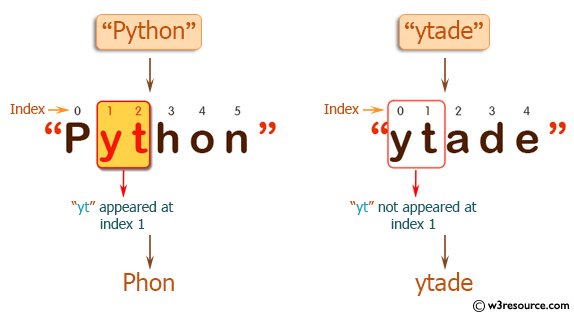
Flowchart:
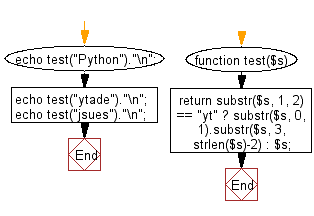
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
Next: Write a PHP program to check the largest number among three given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics