PHP Exercises: Check whether two given integer values are in the range 20..50 inclusive
Write a PHP program to check whether two given integer values are in the range 20..50 inclusive. Return true if one or other is in the said range otherwise false.
Sample Solution:
PHP Code :
<?php
// Define a function that checks if at least one of the given integer values is in the range 20 to 50 inclusive
function test($x, $y)
{
// Check if either $x or $y is within the range [20, 50] inclusive
return ($x >= 20 && $x <= 50) || ($y >= 20 && $y <= 50);
}
// Test the function with various pairs of values
var_dump(test(20, 84)); // true: 20 is within the range
var_dump(test(14, 50)); // true: 50 is within the range
var_dump(test(11, 45)); // true: 45 is within the range
var_dump(test(25, 40)); // true: both 25 and 40 are within the range
var_dump(test(10, 19)); // false: neither 10 nor 19 is within the range
?>
Explanation:
- Function Definition:
- The test function takes two parameters, $x and $y.
- Range Check:
- The function checks if either $x or $y falls within the range [20, 50] (inclusive).
- If at least one of the values is within this range, it returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: test(20, 84)
- $x is 20, which is within the range [20, 50], so it returns true.
- Second Call: test(14, 50)
- $y is 50, which is within the range [20, 50], so it returns true.
- Third Call: test(11, 45)
- $y is 45, which is within the range [20, 50], so it returns true.
- Fourth Call: test(25, 40)
- Both $x and $y (25 and 40) are within the range [20, 50], so it returns true.
- Fifth Call: test(10, 19)
- Neither $x nor $y is within the range [20, 50], so it returns false.
Output:
bool(true) bool(true) bool(true) bool(true) bool(false)
Flowchart:
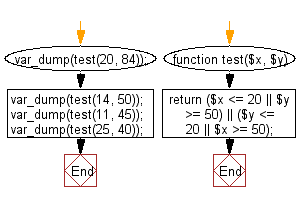
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
Next: Write a PHP program to check if a string 'yt' appears at index 1 in a given string. If it appears return a string without 'yt' otherwise return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics