PHP Exercises: Check two given integers whether either of them is in the range 100..200 inclusive
PHP Basic Algorithm: Exercise-14 with Solution
Write a PHP program to check two given integers whether either of them is in the range 100..200 inclusive.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters: $x and $y
function test($x, $y)
{
// Check if $x is between 100 and 200 (inclusive) or if $y is between 100 and 200 (inclusive)
return ($x >= 100 && $x <= 200) || ($y >= 100 && $y <= 200);
}
// Call the test function with arguments 100 and 199, and var_dump the result
var_dump(test(100, 199));
// Call the test function with arguments 250 and 300, and var_dump the result
var_dump(test(250, 300));
// Call the test function with arguments 105 and 190, and var_dump the result
var_dump(test(105, 190));
?>
Explanation:
- Function Definition:
- The test function takes two parameters: $x and $y.
- Condition Check:
- The function checks if:
- $x is between 100 and 200 (inclusive), or
- $y is between 100 and 200 (inclusive).
- If either $x or $y meets this condition, the function returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: test(100, 199)
- $x is 100, which is within the range [100, 200], so it returns true.
- Second Call: test(250, 300)
- Neither $x nor $y is within the range [100, 200], so it returns false.
- Third Call: test(105, 190)
- Both $x and $y are within the range [100, 200], so it returns true.
- First Call: test(100, 199)
Output:
bool(true) bool(false) bool(true)
Visual Presentation:
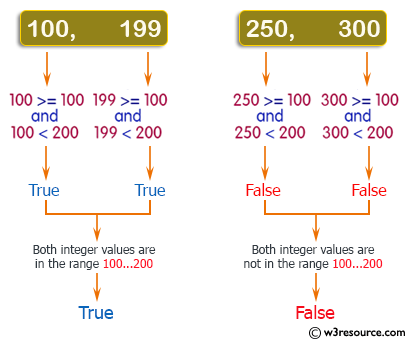
Flowchart:
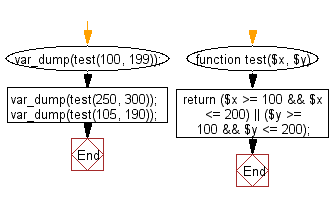
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if one given temperatures is less than 0 and the other is greater than 100.
Next: Write a PHP program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics