PHP Exercises: Create a new array after replacing all the values 5 with 0 shifting all zeros to right direction
128. Replace 5 with 0 and Shift Zeros to Right
Write a PHP program to create a new array after replacing all the values 5 with 0 shifting all zeros to right direction.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Get the size of the input array
$size = sizeof($numbers);
// Initialize variables: $index to keep track of the position in the new array, and $arra1 as an array filled with zeros
$index = 0;
$arra1 = array_fill(0, $size, 0);
// Iterate through the elements of the input array using a for loop
for ($i = 0; $i < $size; $i++)
{
// Check if the current element is not equal to '5'
if ($numbers[$i] != 5)
{
// Assign the current element to the next position in the new array and increment $index
$arra1[$index] = $numbers[$i];
$index++;
}
}
// Return the new array with '5' removed
return $arra1;
}
// Call the 'test' function with an example array and store the result in the variable 'result'
$result = test([1, 2, 5, 3, 5, 7, 5, 9, 11] );
// Print the result array as a string
echo "New array: " . implode(',', $result);
?>
Sample Output:
New array: 1,2,3,7,9,11,0,0,0
Flowchart:
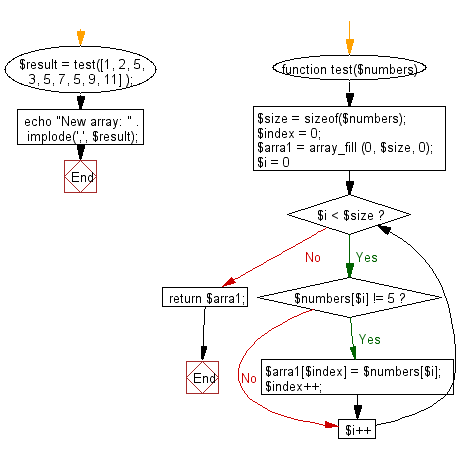
For more Practice: Solve these Related Problems:
- Write a PHP script to substitute all occurrences of 5 in an array with 0 and then shift all zeros to the right end.
- Write a PHP function to first replace any 5's with 0's and then reorder the array so that zeros appear at the end.
- Write a PHP program to process an array by performing two steps: replacement of 5 with 0, and then array reordering to push zeros rightward.
- Write a PHP script to use filtering and merging to replace 5 with 0 and reassemble the array with zeros at the tail.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new array from a given array of integers shifting all zeros to left direction.
Next: Write a PHP program to create new array from a given array of integers shifting all even numbers before all odd numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.