PHP Exercises: Check if a given string starts with 'C#' or not
Write a PHP program to check if a given string starts with 'C#' or not.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $str
function test($str)
{
// Check if the length of $str is less than 3 and it is equal to "C#",
// or if the first two characters of $str are "C#" and the third character is a space.
return (strlen($str) < 3 && $str == "C#") || (substr($str, 0, 2) == "C#" && substr($str, 2, 1) == ' ');
}
// Call the test function with argument "C# Sharp" and var_dump the result
var_dump(test("C# Sharp"));
// Call the test function with argument "C#" and var_dump the result
var_dump(test("C#"));
// Call the test function with argument "C++" and var_dump the result
var_dump(test("C++"));
?>
Explanation:
- Function Definition:
- The test function takes a single parameter, $str.
- Condition Check:
- The function checks if $str meets either of the following conditions:
- $str has fewer than 3 characters and is exactly equal to "C#" (e.g., if $str is "C#").
- The first two characters of $str are "C#" and the third character is a space (e.g., if $str is "C# Sharp").
- If either condition is true, the function returns true; otherwise, it returns false.
- Function Calls and Output:
- First Call: test("C# Sharp")
- The string starts with "C#" and has a space as the third character, so it returns true.
- Second Call: test("C#")
- The string is exactly "C#", which matches the first condition, so it returns true.
- Third Call: test("C++")
- Neither condition is met (it’s neither "C#" nor starts with "C# "), so it returns false.
- First Call: test("C# Sharp")
Output:
bool(true) bool(true) bool(false)
Visual Presentation:
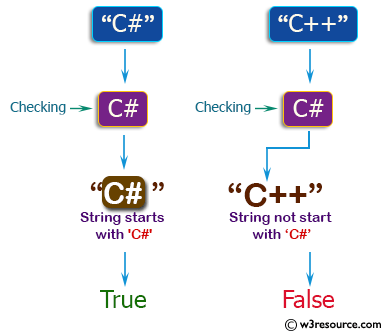
Flowchart:
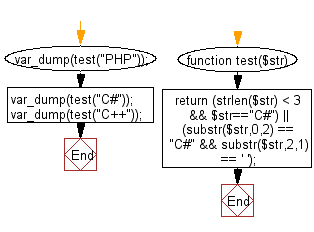
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back. If the given string length is less than 3, use whatever characters are there.
Next: Write a PHP program to check if one given temperatures is less than 0 and the other is greater than 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics