PHP Exercises: Check a given array of integers and return true if the given array contains two 5's next to each other, or two 5 separated by one element
PHP Basic Algorithm: Exercise-117 with Solution
Write a PHP program to check a given array of integers and return true if the given array contains two 5's next to each other, or two 5 separated by one element.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($numbers)
{
// Calculate the length of the array
$len = sizeof($numbers);
// Iterate through the elements of the array up to the second-to-last element
for ($i = 0; $i < $len - 1; $i++)
{
// Check if there are consecutive occurrences of 5 and 5
if ($numbers[$i] == 5 && $numbers[$i + 1] == 5) return true;
// Check if there are non-consecutive occurrences of 5 and 5 with one element in between
if ($i + 2 < $len && $numbers[$i] == 5 && $numbers[$i + 2] == 5) return true;
}
// Return false if there are no consecutive or non-consecutive occurrences of 5 and 5
return false;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([5, 5, 1, 5, 5]));
var_dump(test([1, 2, 3, 4]));
var_dump(test([3, 3, 5, 5, 5, 5]));
var_dump(test([1, 5, 5, 7, 8, 10]));
?>
Sample Output:
bool(true) bool(false) bool(true) bool(true)
Flowchart:
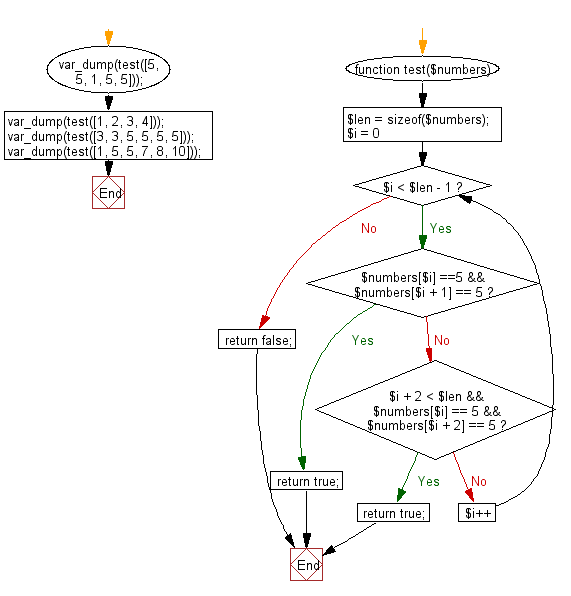
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if an array of integers contains a 3 next to a 3 or a 5 next to a 5 or both.
Next: Write a PHP program to check a given array of integers and return true if there is a 3 with a 5 somewhere later in the given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics