PHP Exercises: Check if a given array of integers contains a 3 or a 5
PHP Basic Algorithm: Exercise-114 with Solution
Write a PHP program to check if a given array of integers contains a 3 or a 5.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is not equal to 3 and not equal to 5, return false if true
if ($nums[$i] != 3 && $nums[$i] != 5) return false;
}
// Return true if all elements are either 3 or 5, otherwise return false
return true;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([5, 5, 5, 5, 5]));
var_dump(test([3, 3, 3, 3]));
var_dump(test([3, 3, 3, 5, 5, 5]));
var_dump(test([1, 6, 8, 10]));
?>
Sample Output:
bool(true) bool(true) bool(true) bool(false)
Flowchart:
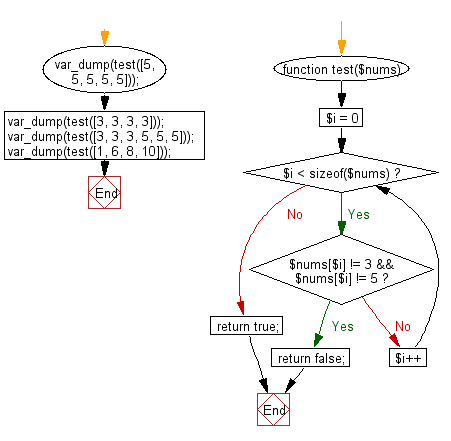
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if the number of 3's is greater than the number of 5's.
Next: Write a PHP program to check if a given array of integers contains no 3 or a 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics