PHP Exercises: Check if the number of 3's is greater than the number of 5's
113. Compare Count of 3's and 5's in Array
Write a PHP program to check if the number of 3's is greater than the number of 5's.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize variables $no_3 and $no_5 with values of 0
$no_3 = 0;
$no_5 = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is equal to 3, increment $no_3 if true
if ($nums[$i] == 3) $no_3++;
// Check if the current element is equal to 5, increment $no_5 if true
if ($nums[$i] == 5) $no_5++;
}
// Return true if the count of 3's is greater than the count of 5's, otherwise return false
return $no_3 > $no_5;
}
// Use 'var_dump' to print the result of calling 'test' with different arrays
var_dump(test([1, 5, 6, 9, 3, 3]));
var_dump(test([1, 5, 5, 5, 10, 17]));
var_dump(test([1, 3, 3, 5, 5, 5]));
?>
Sample Output:
bool(true) bool(false) bool(false)
Flowchart:
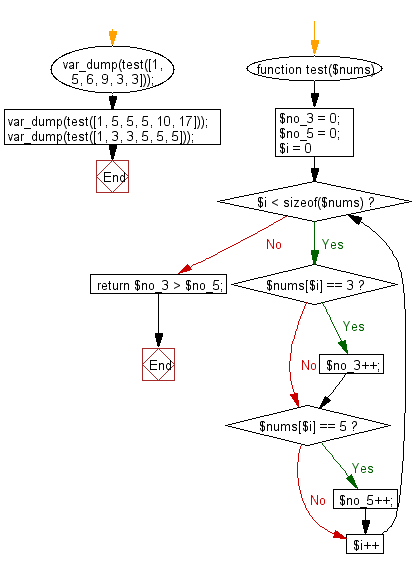
For more Practice: Solve these Related Problems:
- Write a PHP script to count the frequency of the numbers 3 and 5 in an array and return true if the count of 3's exceeds that of 5's.
- Write a PHP function to tally occurrences of 3 and 5 in an array and compare their counts.
- Write a PHP program to implement a counter for specific digits in an array and output a boolean based on the count comparison.
- Write a PHP script to use a loop or array_count_values() to determine whether the number 3 appears more frequently than the number 5.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
Next: Write a PHP program to check if a given array of integers contains a 3 or a 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.