PHP Exercises: Compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6
PHP Basic Algorithm: Exercise-109 with Solution
Write a PHP program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by atleast one 6. Return 0 if the given array has no integer.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize variables $sum, $inSection, and $flag with values of 0
$sum = 0;
$inSection = 0;
$flag = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Set $inSection to 0 at the beginning of each iteration
$inSection = 0;
// Check if the current element is equal to 5
if ($nums[$i] == 5)
{
// Set $inSection to 0 and $flag to 1 if true
$inSection = 0;
$flag = 1;
}
// Check if $inSection is 0 and the current element is 6
if ($inSection == 0 && $nums[$i] == 6)
{
// Check if $flag is 1
if ($flag == 1)
{
// Subtract 5 from $sum, set $inSection to 1
$sum = $sum - 5;
$inSection = 1;
}
// Set $flag to 0
$flag = 0;
}
// Check if $inSection is 0
if ($inSection == 0)
{
// Add the value of the current element to $sum
$sum += $nums[$i];
}
}
// Return the sum of the numbers in the array, excluding those starting with 5 followed by at least one 6
return $sum;
}
// Print the results for different arrays
echo "Sum of the numbers of the said array except those numbers starting with 5 followed by at least one 6:\n". test([1, 5, 7, 9, 10, 17] );
echo "\nSum of the numbers of the said array except those numbers starting with 5 followed by at least one 6:\n". test([1, 5, 6, 9, 10, 17] );
echo "\nSum of the numbers of the said array except those numbers starting with 5 followed by at least one 6:\n". test([5, 6, 7, 9, 10, 17, 1] );
echo "\nSum of the numbers of the said array except those numbers starting with 5 followed by at least one 6:\n". test([11, 9, 10, 17, 5, 6] );
?>
Sample Output:
Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 49 Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 37 Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 44 Sum of the numbers of the said array except those numbers starting with 5 followed by atleast one 6: 47
Flowchart:
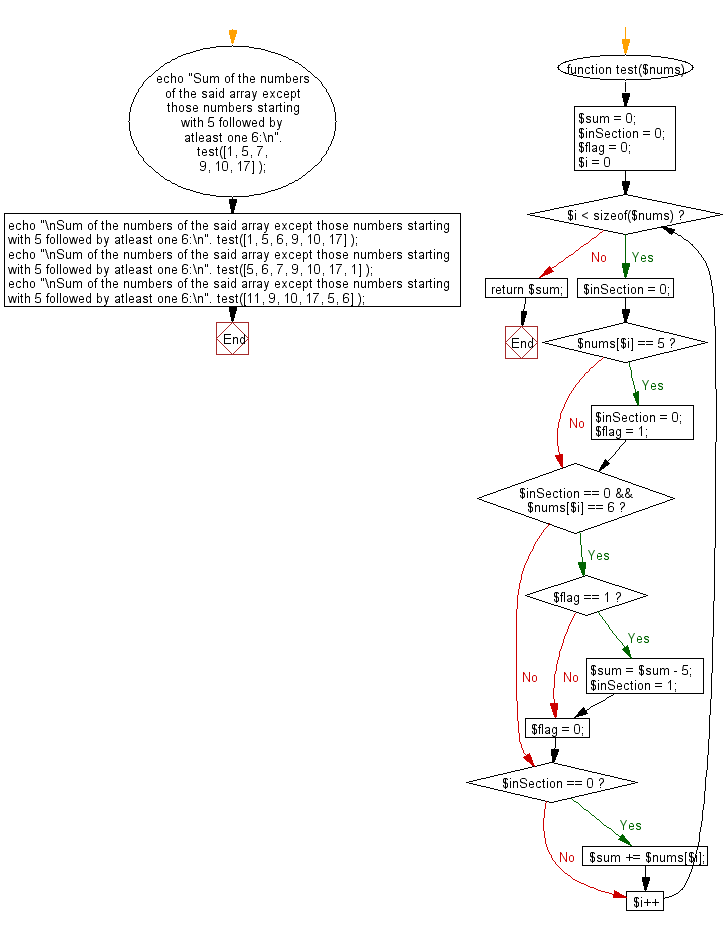
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
Next: Write a PHP program to check if a given array of integers contains 5 next to a 5 somewhere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-109.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics