PHP Exercises: Compute the difference between the largest and smallest values in a given array of integers and length one or more
Write a PHP program to compute the difference between the largest and smallest values in a given array of integers and length one or more.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize variables $small_num and $biggest_num with values of 0
$small_num = 0;
$biggest_num = 0;
// Check if the array has elements (size greater than 0)
if (sizeof($nums) > 0)
// If true, set both $small_num and $biggest_num to the value of the first element in the array
$small_num = $biggest_num = $nums[0];
// Iterate through the elements of the array starting from the second element
for ($i = 1; $i < sizeof($nums); $i++)
{
// Update $small_num to the minimum of its current value and the current array element
$small_num = min($small_num, $nums[$i]);
// Update $biggest_num to the maximum of its current value and the current array element
$biggest_num = max($biggest_num, $nums[$i]);
}
// Return the difference between the largest and smallest values in the array
return $biggest_num - $small_num;
}
// Call the 'test' function with an array [1, 5, 7, 9, 10, 12] and print the result
echo "Difference between the largest and smallest values: ". test([1, 5, 7, 9, 10, 12] );
?>
Sample Output:
Difference between the largest and smallest values: 11
Flowchart:
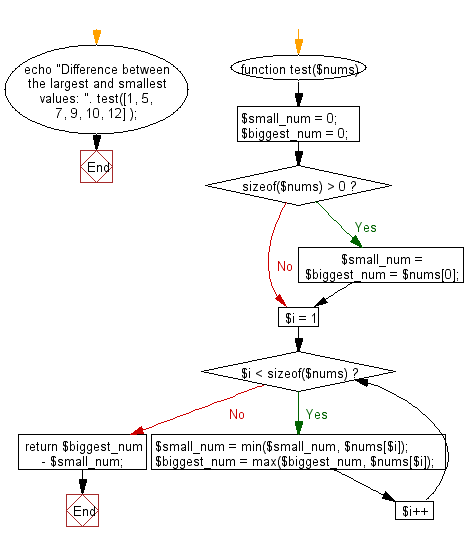
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to count even number of elements in a given array of integers.
Next: Write a PHP program to compute the sum of values in a given array of integers except the number 17. Return 0 if the given array has no integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics