PHP Exercises: Count even number of elements in a given array of integers
106. Count Even Numbers in Array
Write a PHP program to count even number of elements in a given array of integers.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Initialize a variable named $evens with a value of 0
$evens = 0;
// Iterate through the elements of the array using a for loop
for ($i = 0; $i < sizeof($nums); $i++)
{
// Check if the current element is even (divisible by 2), increment $evens if true
if ($nums[$i] % 2 == 0) $evens++;
}
// Return the count of even elements in the array
return $evens;
}
// Call the 'test' function with an array [1, 5, 7, 9, 10, 12] and print the result
echo "Number of even elements: ". test([1, 5, 7, 9, 10, 12] );
?>
Sample Output:
Number of even elements: 2
Flowchart:
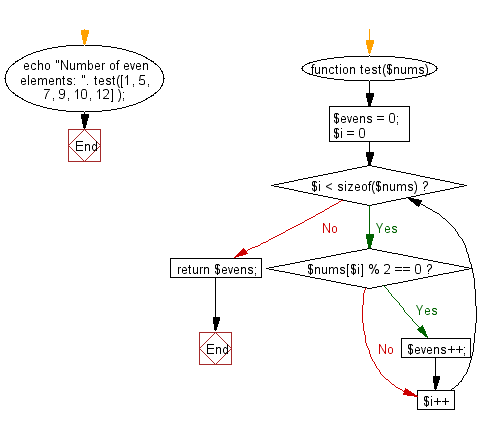
For more Practice: Solve these Related Problems:
- Write a PHP script to iterate over an array and count the number of even integers using a loop.
- Write a PHP function to filter an array for even numbers and then return the count of filtered elements.
- Write a PHP program to use array_filter() to create an array of even numbers and then count them.
- Write a PHP script to compute the count of even numbers in an array without using built-in filtering functions.
Go to:
PREV : New Array from First Two Elements.
NEXT : Difference Between Largest and Smallest.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.