PHP Exercises: Compute the sum of the two given integer values
PHP Basic Algorithm: Exercise-1 with Solution
Write a PHP program to compute the sum of the two given integer values. If the two values are the same, then returns triple their sum.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes two parameters, $x and $y
function test($x, $y)
{
// Use the ternary operator to check if $x is equal to $y
// If true, return the result of multiplying the sum of $x and $y by 3
// If false, return the sum of $x and $y
return $x == $y ? ($x + $y)*3 : $x + $y;
}
// Call the test function with arguments 1 and 2, and echo the result
echo test(1, 2) . "\n";
// Call the test function with arguments 3 and 2, and echo the result
echo test(3, 2) . "\n";
// Call the test function with arguments 2 and 2, and echo the result
echo test(2, 2) . "\n";
?>
Explanation:
- Function Definition:
- A function named test is defined with two parameters: $x and $y.
- Ternary Operation:
- Inside the function, a ternary operator checks if $x is equal to $y.
- If $x == $y, it returns ( $x + $y ) * 3, which is three times the sum of $x and $y.
- If $x is not equal to $y, it returns $x + $y, simply the sum of $x and $y.
- Function Calls and Output:
- First Call: echo test(1, 2);
- Since 1 is not equal to 2, it outputs 1 + 2 = 3.
- Second Call: echo test(3, 2);
- Since 3 is not equal to 2, it outputs 3 + 2 = 5.
- Third Call: echo test(2, 2);
- Since 2 is equal to 2, it outputs (2 + 2) * 3 = 12.
Output:
3 5 12
Visual Presentation:
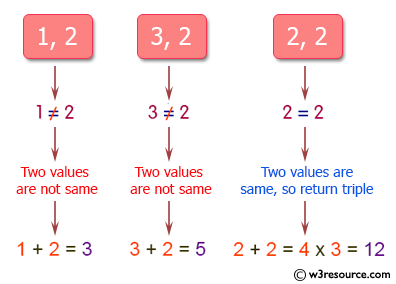
Flowchart:
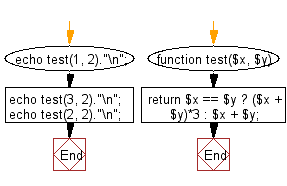
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP Basic Algorithm Exercises Home
Next: Write a PHP program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics