MySQL Date and Time Exercises: Write a query to get first name of employees who joined in 1987
MySQL Date Time: Exercise-17 with Solution
Write a MySQL query to get first name of employees who joined in 1987.
Sample table: employees
Code:
-- This SQL query retrieves the first name and hire date of employees hired in the year 1987.
SELECT
FIRST_NAME, -- Selecting the 'FIRST_NAME' column from the 'employees' table.
HIRE_DATE -- Selecting the 'HIRE_DATE' column from the 'employees' table.
FROM
employees -- Specifying the 'employees' table.
WHERE
YEAR(HIRE_DATE)=1987; -- Filtering the rows to include only those where the hire date year is 1987.
Explanation:
- This SQL query selects the first name and hire date of employees from the 'employees' table.
- The WHERE clause filters the result set to include only records where the year part of the hire date is 1987.
- The YEAR() function extracts the year part from the HIRE_DATE column, and it is compared to 1987 to filter the records.
Sample Output:
FIRST_NAME HIRE_DATE Steven 1987-06-17T04:00:00.000Z Neena 1987-06-18T04:00:00.000Z Lex 1987-06-19T04:00:00.000Z Alexander 1987-06-20T04:00:00.000Z Bruce 1987-06-21T04:00:00.000Z David 1987-06-22T04:00:00.000Z Valli 1987-06-23T04:00:00.000Z Diana 1987-06-24T04:00:00.000Z Nancy 1987-06-25T04:00:00.000Z Daniel 1987-06-26T04:00:00.000Z John 1987-06-27T04:00:00.000Z Ismael 1987-06-28T04:00:00.000Z Jose Manuel 1987-06-29T04:00:00.000Z Luis 1987-06-30T04:00:00.000Z Den 1987-07-01T04:00:00.000Z Alexander 1987-07-02T04:00:00.000Z Shelli 1987-07-03T04:00:00.000Z Sigal 1987-07-04T04:00:00.000Z Guy 1987-07-05T04:00:00.000Z Karen 1987-07-06T04:00:00.000Z Matthew 1987-07-07T04:00:00.000Z Adam 1987-07-08T04:00:00.000Z Payam 1987-07-09T04:00:00.000Z Shanta 1987-07-10T04:00:00.000Z Kevin 1987-07-11T04:00:00.000Z Julia 1987-07-12T04:00:00.000Z Irene 1987-07-13T04:00:00.000Z James 1987-07-14T04:00:00.000Z Steven 1987-07-15T04:00:00.000Z Laura 1987-07-16T04:00:00.000Z Mozhe 1987-07-17T04:00:00.000Z James 1987-07-18T04:00:00.000Z TJ 1987-07-19T04:00:00.000Z Jason 1987-07-20T04:00:00.000Z Michael 1987-07-21T04:00:00.000Z Ki 1987-07-22T04:00:00.000Z Hazel 1987-07-23T04:00:00.000Z Renske 1987-07-24T04:00:00.000Z Stephen 1987-07-25T04:00:00.000Z John 1987-07-26T04:00:00.000Z Joshua 1987-07-27T04:00:00.000Z Trenna 1987-07-28T04:00:00.000Z Curtis 1987-07-29T04:00:00.000Z Randall 1987-07-30T04:00:00.000Z Peter 1987-07-31T04:00:00.000Z John 1987-08-01T04:00:00.000Z Karen 1987-08-02T04:00:00.000Z Alberto 1987-08-03T04:00:00.000Z Gerald 1987-08-04T04:00:00.000Z Eleni 1987-08-05T04:00:00.000Z Peter 1987-08-06T04:00:00.000Z David 1987-08-07T04:00:00.000Z Peter 1987-08-08T04:00:00.000Z Christopher 1987-08-09T04:00:00.000Z Nanette 1987-08-10T04:00:00.000Z Oliver 1987-08-11T04:00:00.000Z Janette 1987-08-12T04:00:00.000Z Patrick 1987-08-13T04:00:00.000Z Allan 1987-08-14T04:00:00.000Z Lindsey 1987-08-15T04:00:00.000Z Louise 1987-08-16T04:00:00.000Z Sarath 1987-08-17T04:00:00.000Z Clara 1987-08-18T04:00:00.000Z Danielle 1987-08-19T04:00:00.000Z Mattea 1987-08-20T04:00:00.000Z David 1987-08-21T04:00:00.000Z Sundar 1987-08-22T04:00:00.000Z Amit 1987-08-23T04:00:00.000Z Lisa 1987-08-24T04:00:00.000Z Harrison 1987-08-25T04:00:00.000Z Tayler 1987-08-26T04:00:00.000Z William 1987-08-27T04:00:00.000Z Elizabeth 1987-08-28T04:00:00.000Z Sundita 1987-08-29T04:00:00.000Z Ellen 1987-08-30T04:00:00.000Z Alyssa 1987-08-31T04:00:00.000Z Jonathon 1987-09-01T04:00:00.000Z Jack 1987-09-02T04:00:00.000Z Kimberely 1987-09-03T04:00:00.000Z Charles 1987-09-04T04:00:00.000Z Winston 1987-09-05T04:00:00.000Z Jean 1987-09-06T04:00:00.000Z Martha 1987-09-07T04:00:00.000Z Girard 1987-09-08T04:00:00.000Z Nandita 1987-09-09T04:00:00.000Z Alexis 1987-09-10T04:00:00.000Z Julia 1987-09-11T04:00:00.000Z Anthony 1987-09-12T04:00:00.000Z Kelly 1987-09-13T04:00:00.000Z Jennifer 1987-09-14T04:00:00.000Z Timothy 1987-09-15T04:00:00.000Z Randall 1987-09-16T04:00:00.000Z Sarah 1987-09-17T04:00:00.000Z Britney 1987-09-18T04:00:00.000Z Samuel 1987-09-19T04:00:00.000Z Vance 1987-09-20T04:00:00.000Z Alana 1987-09-21T04:00:00.000Z Kevin 1987-09-22T04:00:00.000Z Donald 1987-09-23T04:00:00.000Z Douglas 1987-09-24T04:00:00.000Z Jennifer 1987-09-25T04:00:00.000Z Michael 1987-09-26T04:00:00.000Z Pat 1987-09-27T04:00:00.000Z Susan 1987-09-28T04:00:00.000Z Hermann 1987-09-29T04:00:00.000Z Shelley 1987-09-30T04:00:00.000Z William 1987-10-01T04:00:00.000Z
Pictorial Presentation of the above query:
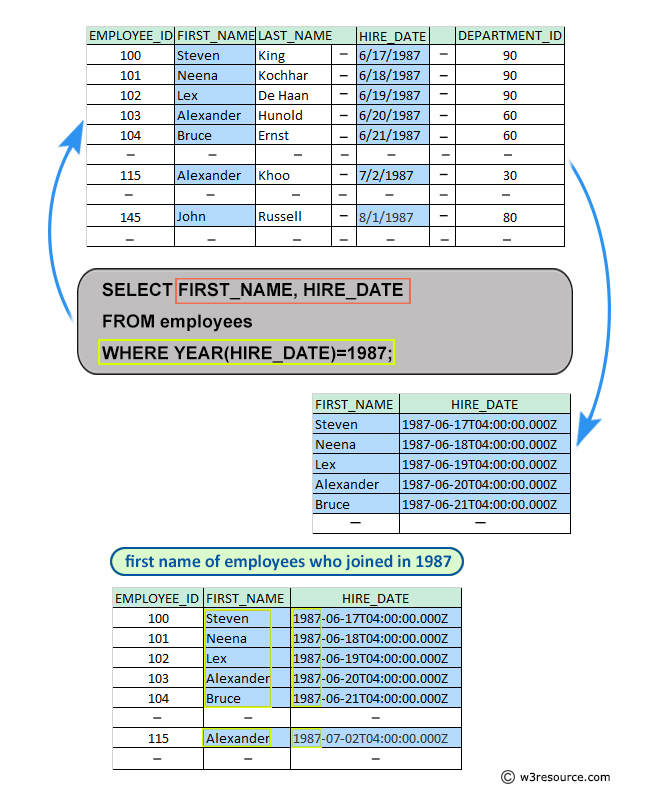
MySQL Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a MySQL query to get the years in which more than 10 employees joined.
Next:Write a MySQL query to get department name, manager name, and salary of the manager for all managers whose experience is more than 5 years.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics