JavaScript Exercises: Remove duplicates from a given stack
JavaScript Stack: Exercise-9 with Solution
Remove Duplicates from Stack
Write a JavaScript program to remove duplicates from a given stack.
Sample Solution:
JavaScript Code:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty())
return "Underflow";
return this.items.pop();
}
peek() {
if (this.isEmpty())
return "No elements in Stack";
return this.items[this.items.length - 1];
}
isEmpty() {
return this.items.length === 0;
}
removeDuplicates() {
const uniqueItems = [];
const seen = new Set();
for (let i = 0; i < this.items.length; i++) {
const currentItem = this.items[i];
if (!seen.has(currentItem)) {
uniqueItems.push(currentItem);
seen.add(currentItem);
}
}
this.items = uniqueItems;
}
displayStack(stack) {
console.log("Stack elements are:");
let str = "";
for (let i = 0; i < stack.items.length; i++)
str += stack.items[i] + " ";
return str.trim();
}
}
console.log("Initialize a stack:")
let stack = new Stack();
console.log("Input some elements on the stack:")
stack.push(1);
stack.push(6);
stack.push(3);
stack.push(2);
stack.push(5);
stack.push(6);
console.log(stack.displayStack(stack));
stack.removeDuplicates();
console.log("After removing duplicates from the said stack:");
console.log(stack.displayStack(stack));
console.log("Input two more elements on the stack:")
stack.push(1);
stack.push(6);
console.log(stack.displayStack(stack));
stack.removeDuplicates();
console.log("After removing duplicates from the said stack:");
console.log(stack.displayStack(stack));
Sample Output:
Initialize a stack: Input some elements on the stack: Stack elements are: 1 6 3 2 5 6 After removing duplicates from the said stack: Stack elements are: 1 6 3 2 5 Input two more elements on the stack: Stack elements are: 1 6 3 2 5 1 6 After removing duplicates from the said stack: Stack elements are: 1 6 3 2 5
Flowchart:
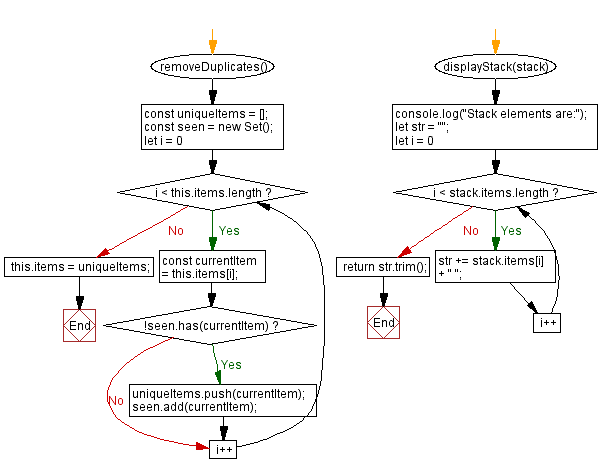
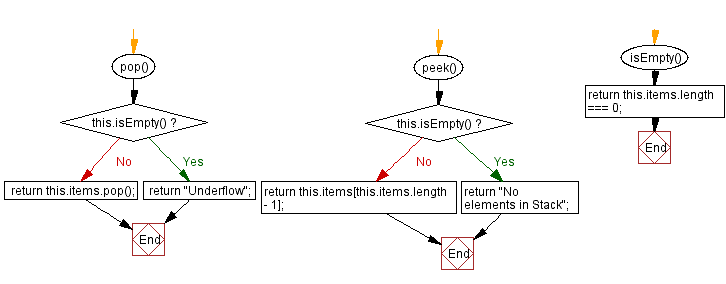
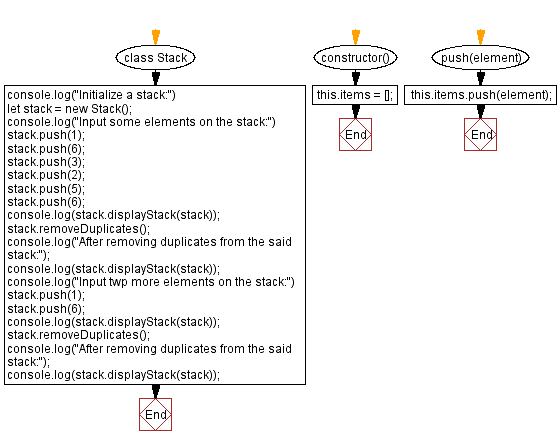
Live Demo:
See the Pen javascript-stack-exercise-8 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that removes duplicate elements from a stack and retains only the first occurrence of each item.
- Write a JavaScript function that uses an auxiliary stack to filter out duplicate values while preserving order.
- Write a JavaScript function that iterates over a stack and builds a new stack free of duplicate entries.
- Write a JavaScript function that checks for duplicates in a stack using a hash table and removes them in a single pass.
Improve this sample solution and post your code through Disqus
Stack Previous: Check if an element is present or not in a stack.
Stack Exercises Next: Top and bottom elements of a stack.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics