JavaScript Sorting Algorithm: Find the highest index at which a value should be inserted into an array in order to maintain its sort order, based on a provided iterator function
JavaScript Sorting Algorithm: Exercise-17 with Solution
Find Highest Insert Index
Write a JavaScript program to find the highest index at which a value should be inserted into an array to maintain its sort order. This is based on a provided iterator function.
Sample Solution:
JavaScript Code:
const sortedLastIndexBy = (arr, n, fn) => {
const isDescending = fn(arr[0]) > fn(arr[arr.length - 1]);
const val = fn(n);
const index = arr
.map(fn)
.reverse()
.findIndex(el => (isDescending ? val <= el : val >= el));
return index === -1 ? 0 : arr.length - index;
};
console.log(sortedLastIndexBy([{ x: 4 }, { x: 5 }], { x: 4 }, o => o.x));
Sample Output:
1
Flowchart:
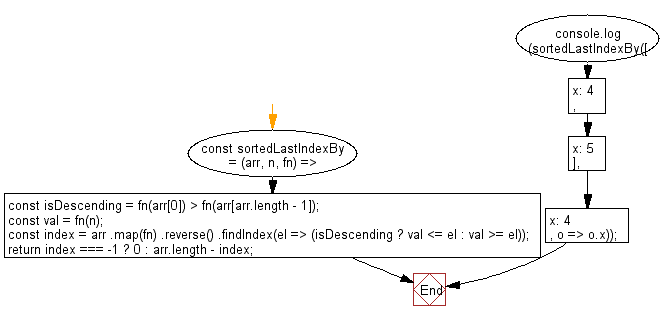
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the highest index at which a given value should be inserted in a sorted array.
- Write a JavaScript function that uses binary search to efficiently determine the highest insert index.
- Write a JavaScript function that handles duplicate values by returning the last valid insertion point.
- Write a JavaScript function that validates the sorted array and the target value before computing the index.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to sort an array of objects, ordered by properties and orders.
Next: Write a JavaScript program to sort an array of objects, ordered by a property, based on the array of orders provided.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.