JavaScript Sorting Algorithm: Sort an array of numbers, using the bucket sort algorithm
JavaScript Sorting Algorithm: Exercise-15 with Solution
Bucket Sort
Write a JavaScript program to sort an array of numbers, using the bucket sort algorithm.
- Use Math.min(), Math.max() and the spread operator (...) to find the minimum and maximum values of the given array.
- Use Array.from() and Math.floor() to create the appropriate number of buckets (empty arrays).
- Use Array.prototype.forEach() to populate each bucket with the appropriate elements from the array.
- Use Array.prototype.reduce(), the spread operator (...) and Array.prototype.sort() to sort each bucket and append it to the result.
Sample Solution:
JavaScript Code:
//Source:https://bit.ly/3hEZdCl
// BucketSort
const bucketSort = (arr, size = 5) => {
const min = Math.min(...arr);
const max = Math.max(...arr);
const buckets = Array.from(
{ length: Math.floor((max - min) / size) + 1 },
() => []
);
arr.forEach(val => {
buckets[Math.floor((val - min) / size)].push(val);
});
return buckets.reduce((acc, b) => [...acc, ...b.sort((a, b) => a - b)], []);
};
console.log(bucketSort([6, 3, 4, 1]));
Sample Output:
[1,3,4,6]
Flowchart:
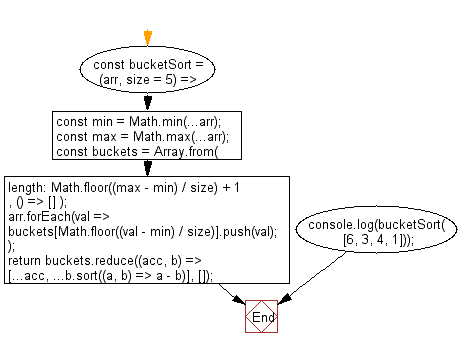
Live Demo:
See the Pen javascript-common-editor by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that implements bucket sort for an array of floating-point numbers between 0 and 1.
- Write a JavaScript function that divides the input array into buckets, sorts each bucket with insertion sort, and merges the results.
- Write a JavaScript function that handles inputs with values outside the expected range by normalizing them.
- Write a JavaScript function that outputs the sorted array and the intermediate bucket distributions for verification.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to sort a list of elements using Bogosort.
Next: Write a JavaScript program to sort an array of objects, ordered by properties and orders.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics