JavaScript Class: Person with name, age, and country properties
JavaScript OOP: Exercise-1 with Solution
Person Class with Details
Write a JavaScript program to create a class called "Person" with properties for name, age and country. Include a method to display the person's details. Create two instances of the 'Person' class and display their details.
Sample Solution:
JavaScript Code:
class Person {
constructor(name, age, country) {
this.name = name;
this.age = age;
this.country = country;
}
displayDetails() {
console.log(`Name: ${this.name}`);
console.log(`Age: ${this.age}`);
console.log(`Country: ${this.country}`);
}
}
// Create instances of the Person class
const person1 = new Person('Francisca Rohan', 25, 'USA');
const person2 = new Person('Raimond Aruna', 30, 'Netherlands');
// Display details of person1
console.log('Person-1 Details:');
person1.displayDetails();
// Display details of person2
console.log('\nPerson-2 Details:');
person2.displayDetails();
Sample Output:
"Person-1 Details:" "Name: Francisca Rohan" "Age: 25" "Country: USA" " Person-2 Details:" "Name: Raimond Aruna" "Age: 30" "Country: Netherlands"
Note: Executed on JS Bin
Explanation:
In the above exercise we create a "Person" class with properties for name, age, and country. It includes a method to display the person's details.
Finally it creates two instances of the Person class and displays their details using the displayDetails() method.
Flowchart:
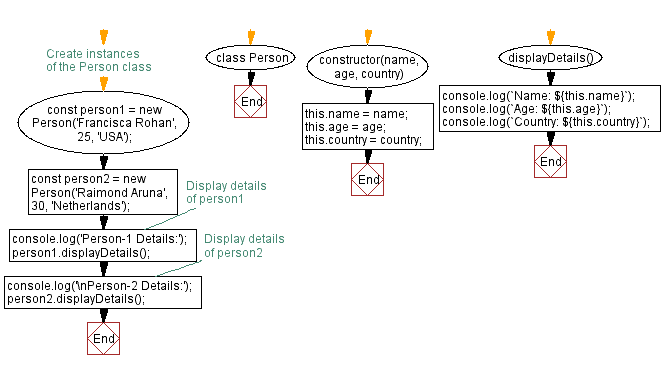
Live Demo:
See the Pen javascript-oop-exercise-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
OOP Exercises Previous: JavaScript OOP Exercises Home.
OOP Exercises Next: Rectangle with area and perimeter methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics