JavaScript: Make capitalize the first letter of each word in a string
JavaScript String: Exercise-9 with Solution
Capitalize Each Word
Write a JavaScript function to capitalize the first letter of each word in a string.
Test Data:
console.log(capitalize_Words('js string exercises'));
"Js String Exercises"
Visual Presentation:
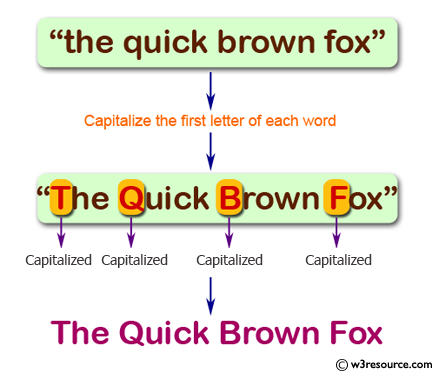
Sample Solution:
JavaScript Code:
// Define a function named capitalize_Words that takes a string str as input
function capitalize_Words(str)
{
// Use the replace method with a regular expression to match words (\w) followed by zero or more non-space characters (\S*)
// For each matched word, use a callback function to capitalize the first character and convert the rest of the characters to lowercase
return str.replace(/\w\S*/g, function(txt){return txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase();});
}
// Call the capitalize_Words function with the argument 'js string exercises' and output the result
console.log(capitalize_Words('js string exercises'));
Output:
Js String Exercises
Explanation:
In the exercise above,
- The function "capitalize_Words()" is defined with a single parameter 'str', representing the input string.
- Within the function, a regular expression (/\w\S*/g) is used with the "replace()" method to match words in the string.
- For each matched word, a callback function is invoked, which capitalizes the first character using 'txt.charAt(0).toUpperCase()' and converts the rest of the characters to lowercase using 'txt.substr(1).toLowerCase()'.
- The "replace()" method replaces each matched word with the modified version (capitalized first letter and lowercase rest).
- Finally, the modified string is returned from the function.
Flowchart:
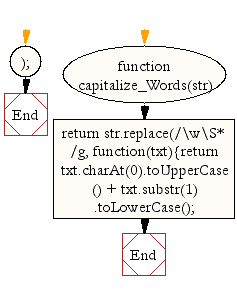
Live Demo:
See the Pen JavaScript Make capitalize the first letter of each word in a string - string-ex-9 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to capitalize the first letter of a string.
Next: Write a JavaScript function that takes a string which has lower and upper case letters as a parameter and converts upper case letters to lower case, and lower case letters to upper case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics