JavaScript: Reverse a Binary string
JavaScript String: Exercise-64 with Solution
Write a JavaScript function that takes a positive integer and reverses the binary representation of that integer. Finally return the decimal version of the binary string.
Test Data:
(100) -> 19
Explanation:
Binary representation of 100 is 1100100
Reverse of 1100100 is 10011
Decimal form of 10011 is 19
(1134) -> 945
Explanation:
Binary representation of 1134 is 10001101110
Reverse of 10001101110 is 1110110001
Decimal form of 1110110001 is 945
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript function to Reverse a Binary string</title>
</head>
<body>
</body>
</html>
JavaScript Code:
function test(n) {
return parseInt(n.toString(2).split('').reverse().join(''), 2);
}
console.log(test(100));
console.log(test(1134));
Sample Output:
19 945
Flowchart:
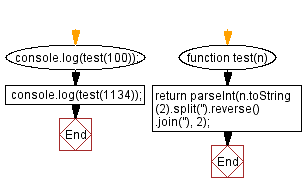
Live Demo:
See the Pen javascript-string-exercise-64 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Longest Palindromic Subsequence.
Next: Javascript regexp Exercises
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.