JavaScript: Reverse words in a given string
JavaScript String: Exercise-60 with Solution
Reverse Words
Write a JavaScript function to reverse words in a given string.
Test Data:
("abc") -> "cba"
("JavaScript Exercises") -> "tpircSavaJ sesicrexE"
(1234) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function named 'test' with a single parameter 'text'
function test(text) {
// Check if the input string is empty
if (text.length === 0)
{
// Return a message if the input string is empty
return 'String should not be empty!'
}
// Check if the input parameter 'text' is not a string
if (typeof text !== 'string')
{
// Return a message if the input is not a string
return 'It must be a string.'
}
// Create an empty array to store words
var words = [];
// Split the input text into an array of words using whitespace as the delimiter
words = text.match(/\S+/g);
// Create an empty string to store the reversed words
var reverse_word = "";
// Iterate through each word in the 'words' array
for (var i = 0; i < words.length; i++) {
// Reverse each word, join the characters, and add it to the 'reverse_word' string
reverse_word += words[i].split('').reverse().join('') + " ";
}
// Return the string with reversed words
return reverse_word
}
// Test the 'test' function with different input strings and output the result
console.log(test("abc")) // 'cba'
console.log(test("JavaScript Exercises")) // 'tpircsexE scirpSavaJ'
console.log(test(1234)) // It must be a string.
Output:
cba tpircSavaJ sesicrexE It must be a string.
Explanation:
In the exercise above,
- The function "test()" is defined, taking a single parameter named 'text'.
- It first checks if the input string is empty. If it is, the function returns a message indicating that the string should not be empty.
- Next, it checks if the input parameter 'text' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating it must be a string.
- If the input is a non-empty string, the function splits the text into an array of words using a regular expression that matches non-whitespace characters.
- It then iterates through each word in the array of words.
- For each word, it splits it into an array of characters. It reverses the array, joins the characters back into a string, and adds it to the 'reverse_word' string with a space.
- After reversing each word, the function returns the string with reversed words.
Flowchart:
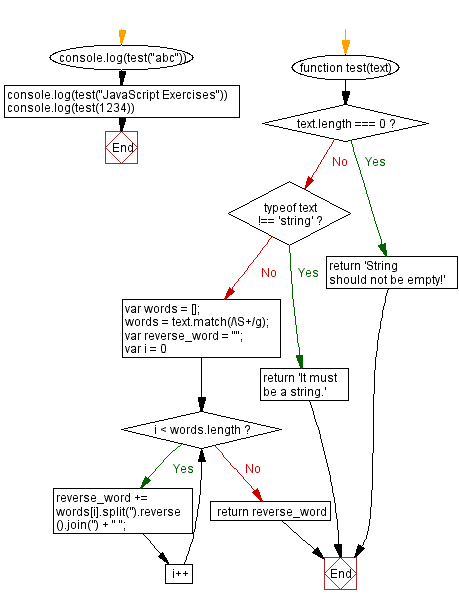
Live Demo:
See the Pen javascript-string-exercise-60 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that reverses the order of words in a given string.
- Write a JavaScript function that splits a sentence into words, reverses each word, and then rejoins them.
- Write a JavaScript function that handles multiple spaces and punctuation correctly when reversing words.
- Write a JavaScript function that validates the input and returns an error if it is not a string.
Improve this sample solution and post your code through Disqus
Previous: Find the most frequent word in a string.
Next: Longest common subsequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.