JavaScript: Hide email addresses to protect from unauthorized user
JavaScript String: Exercise-6 with Solution
Hide Email Address
Write a JavaScript function that hides email addresses to prevent unauthorized access.
Test Data:
console.log(protect_email("[email protected]"));
"[email protected]"
Sample Solution:
JavaScript Code:
// Define a function called protect_email, which takes a user_email string as input
protect_email = function (user_email) {
// Declare variables avg, splitted, part1, and part2
var avg, splitted, part1, part2;
// Split the user_email string into two parts using "@" as the delimiter and store them in the splitted array
splitted = user_email.split("@");
// Store the first part of the email in the part1 variable
part1 = splitted[0];
// Calculate the average length of the first part of the email
avg = part1.length / 2;
// Extract the substring of part1 from index 0 to (part1.length - avg) and store it back in part1
part1 = part1.substring(0, (part1.length - avg));
// Store the second part of the email in the part2 variable
part2 = splitted[1];
// Return a protected version of the email by concatenating part1, "...@", and part2
return part1 + "...@" + part2;
};
// Call the protect_email function with the input "[email protected]" and log the result to the console
console.log(protect_email("[email protected]"));
Output:
[email protected]
Explanation:
In the exercise above,
- The function "protect_email()" splits the input email address into two parts using the "@" symbol as a delimiter and stores them in the 'splitted' array.
- It extracts the first part of the email address from the 'splitted' array and stores it in the 'part1' variable.
- It calculates the average length of 'part1'.
- It extracts a substring from 'part1' starting from index 0 to ('part1.length - avg') and stores it back in the 'part1' variable. This effectively removes characters from the middle of the email address.
- It retrieves the second part of the email address from the 'splitted' array and stores it in the 'part2' variable.
- Finally, it concatenates the modified 'part1', "...@", and 'part2' to form the partially protected email address and returns it.
Flowchart:
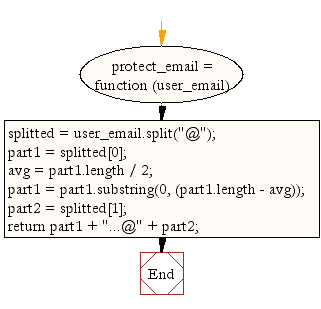
Live Demo:
See the Pen JavaScript Hide email addresses to protect from unauthorized user - string-ex-6 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that obfuscates an email address by masking part of the username with ellipses.
- Write a JavaScript function that splits an email into username and domain, then replaces part of the username with a fixed pattern.
- Write a JavaScript function that validates the email format before hiding its internal characters.
- Write a JavaScript function that dynamically determines the number of characters to mask in an email address.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to convert a string in abbreviated form.
Next: rite a JavaScript function to parameterize a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.