JavaScript: Find the most frequent word in a string
JavaScript String: Exercise-59 with Solution
Most Frequent Word
Write a JavaScript program to find the most frequent word in a given string.
Test Data:
("The quick brown fox jumps over the lazy dog") -> "the"
("Python is a high-level, general-purpose programming language.") -> "python"
(" It was the same man, she was sure of it. It's always the same, Chauncey.") -> "was"
(12321) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function named 'test' with a single parameter 'text'
const test = (text) => {
// Check if the input string is empty
if (text.length === 0)
{
// Return a message if the input string is empty
return 'String should not be empty!'
}
// Check if the input parameter 'text' is not a string
if (typeof text !== 'string')
{
// Return a message if the input is not a string
return 'It must be a string.'
}
// Split the input text into an array of words using space as the delimiter
const data = text.split(' ')
// If there's only one word in the text, return that word
if (data.length < 2) {
return data[0]
}
// Split the input text into an array of words using space as the delimiter
const words = text.split(' ')
// If there's only one word in the text, return that word
if (words.length < 2) {
return words[0]
}
// Create an empty object to store word frequencies
const temp = {}
// Iterate through each word in the array of words
words.forEach(word => {
// Convert each word to lowercase and increment its frequency in the 'temp' object
temp[word.toLocaleLowerCase()] = temp[word.toLocaleLowerCase()] + 1 || 1
})
// Find the word with the maximum frequency in the 'temp' object
const max = Object.keys(temp).reduce((n, word) => {
// If the frequency of the current word is greater than the maximum frequency seen so far, update 'max'
if (temp[word] > n.count)
{
return { word, count: temp[word] }
}
else
{
return n
}
}, { word: '', count: 0 })
// Return the word with the maximum frequency
return max.word
}
// Test the 'test' function with different input strings and output the result
console.log(test("The quick brown fox jumps over the lazy dog")) // 'the'
console.log(test("Python is a high-level, general-purpose programming language.")) // 'a'
console.log(test(" It was the same man, she was sure of it. It's always the same, Chauncey.")) // 'the'
console.log(test(12321)) // It must be a string.
Output:
the python was It must be a string.
Explanation:
In the exercise above,
- The function "test()" is defined using arrow function syntax, taking a single parameter named 'text'.
- It first checks if the input string is empty. If it is, the function returns a message indicating that the string should not be empty.
- Next, it checks if the input parameter 'text' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating it must be a string.
- If the input is a non-empty string, the function splits the text into an array of words using space as the delimiter.
- If there's only one word in the text, the function returns that word.
- It then creates an empty object to store word frequencies and iterates through each word in the array of words.
- For each word, it converts it to lowercase and increments its frequency in the object.
- After counting the frequencies of each word, the function finds the word with the maximum frequency by iterating through the object's entries.
Flowchart:
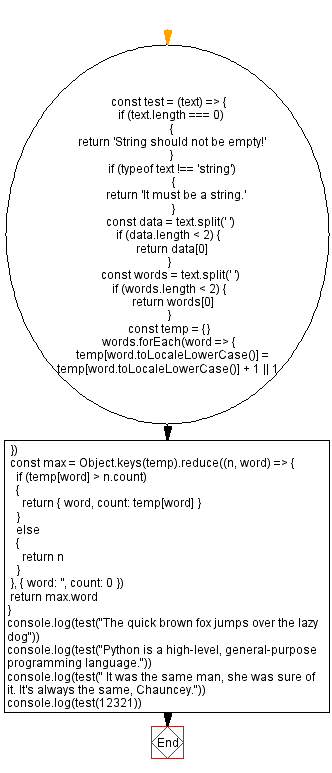
Live Demo:
See the Pen javascript-string-exercise-59 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that splits a string into words and finds the most frequently occurring word using a frequency counter.
- Write a JavaScript function that converts the string to lowercase before counting word occurrences for case-insensitivity.
- Write a JavaScript function that handles punctuation by stripping non-alphanumeric characters prior to word counting.
- Write a JavaScript function that returns a message indicating the most frequent word and its count.
Improve this sample solution and post your code through Disqus.
Previous: Find the most frequent character in a string.
Next: Reverse words in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.