JavaScript: Find the most frequent character in a string
JavaScript String: Exercise-58 with Solution
Most Frequent Character
Write a JavaScript program to find the most frequent character in a given string.
Test Data:
("Madam") -> "a"
("civic") -> "c"
("HellloL223LLL") -> "L"
(12321) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function named 'test' with a single parameter 'str'
const test = (str) => {
// Check if the input string is empty
if (str.length === 0)
{
// Return a message if the input string is empty
return 'String should not be empty!'
}
// Check if the input parameter 'str' is not a string
if (typeof str !== 'string')
{
// Return a message if the input is not a string
return 'It must be a string.'
}
// Create a Map to store the occurrences of each character in the string
const occurrence_Map = new Map()
// Iterate through each character in the string
for (const char of str)
{
// Increment the occurrence count of the current character in the Map, or set it to 1 if it doesn't exist
occurrence_Map.set(char, occurrence_Map.get(char) + 1 || 1)
}
// Find the character with the maximum occurrence count in the occurrence Map
let max_char = { char: '', occur: -Infinity }
for (const [char, occur] of occurrence_Map) {
// Update max_char if the occurrence of the current character is greater than the current maximum occurrence
if (occur > max_char.occur) {
max_char = { char,occur }
}
}
// Return the character with the maximum occurrence count
return max_char.char
}
// Test the 'test' function with different input strings and output the result
console.log(test("Madam")) // 'a'
console.log(test("civic")) // 'c'
console.log(test("HellloL223LLL")) // 'L'
console.log(test(12321)) // It must be a string.
Output:
a c L It must be a string.
Explanation:
In the exercise above,
- The function "test()" is defined using arrow function syntax, taking a single parameter named 'str'.
- It first checks if the input string is empty. If it is, the function returns a message indicating that the string should not be empty.
- Next, it checks if the input parameter 'str' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating it must be a string.
- If the input is a non-empty string, the function counts the occurrences of each character in the string using a 'Map' data structure. It iterates through each character in the string and updates the occurrence count in the Map.
- After counting the occurrences of each character, the function finds the character with the maximum occurrence count in the 'Map' by iterating through its entries.
- Finally, the function returns the character with the maximum occurrence count.
Flowchart:
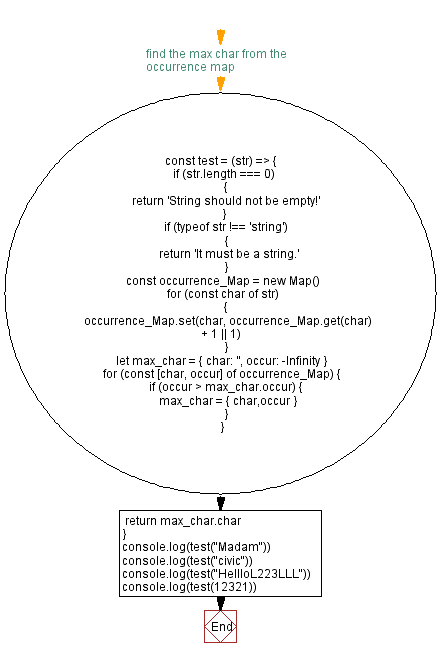
Live Demo:
See the Pen javascript-string-exercise-58 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that finds the most frequent character in a string using a frequency map.
- Write a JavaScript function that handles ties by returning the first most frequent character.
- Write a JavaScript function that converts the string to a uniform case before computing frequency.
- Write a JavaScript function that validates the input type and returns an error if the input is not a string.
Improve this sample solution and post your code through Disqus.
Previous: Rearrange a string to become a palindrome.
Next: Find the most frequent word in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.