JavaScript: Check a string is in Pascal case
JavaScript String: Exercise-56 with Solution
Check Pascal Case
A PascalCase naming convention capitalizes the first letter of each compound word in a variable. It is a best practice in software development to use descriptive variable names.
Write a JavaScript function to check whether a string is in Pascal case or not.
Test Data:
("XmlStream") -> true
("IOStream") -> true
("javascript") -> false
(12356) -> "It must be a string."
Sample Solution:
JavaScript Code:
// Define a function named 'test' with a single parameter 'word'
const test = (word) => {
// Check if the input parameter 'word' is not a string
if (typeof word !== 'string')
{
// Return a message if the input is not a string
return 'It must be a string.'
}
// Define a regular expression pattern to match words starting with an uppercase letter followed by zero or more letters (uppercase or lowercase)
const pattern = /^[A-Z][A-Za-z]*$/
// Test if the input word matches the defined pattern
return pattern.test(word)
}
// Test the 'test' function with different input strings and output the result
console.log(test("XmlStream")) // true
console.log(test("IOStream")) // true
console.log(test("IEnumerable")) // true
console.log(test("javascript")) // false
console.log(test(12356)) // It must be a string.
Output:
true true true false It must be a string.
Explanation:
In the exercise above,
- The function "test()" is defined using arrow function syntax, taking a single parameter named 'word'.
- It checks if the input parameter 'word' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating that it must be a string.
- If the input is a string, the function defines a regular expression pattern (/^[A-Z][A-Za-z]*$/) to match words that start with an uppercase letter followed by zero or more letters (uppercase or lowercase).
- The function then tests if the input word matches the defined pattern using the "test()" method of the regular expression object.
- The function returns 'true' if the word matches the pattern and 'false' otherwise.
Flowchart:
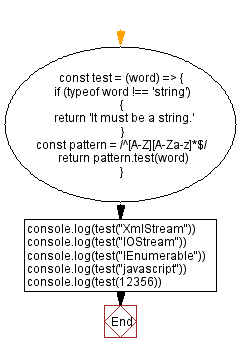
Live Demo:
See the Pen javascript-string-exercise-56 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a string is in PascalCase by verifying each word starts with an uppercase letter and no spaces exist.
- Write a JavaScript function that uses regular expressions to test for PascalCase format in a string.
- Write a JavaScript function that validates the input type and returns a boolean indicating PascalCase compliance.
- Write a JavaScript function that converts a string to PascalCase and then compares it to the original string for equality.
Improve this sample solution and post your code through Disqus.
Previous: Check a string is a Pangram or not.
Next: Rearrange a string to become a palindrome.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.