JavaScript: Check a string is a Pangram or not
JavaScript String: Exercise-55 with Solution
Pangram Check
A pangram or holoalphabetic sentence is a sentence using every letter of a given alphabet at least once. Pangrams have been used to display typefaces, test equipment, and develop skills in handwriting, calligraphy, and keyboarding.
Write a JavaScript function to check whether a string is a Pangram or not.
Example : "The quick brown fox jumps over the lazy dog"
Test Data:
("The quick brown fox jumps over the lazy dog") -> true
("Waltz, bad nymph, for quick jigs vex.") -> true
("The five boxing wizards jump quickly.") -> true
("The boxing wizards jump quickly.") -> false
(12356) -> "It must be a string."
Sample Solution:
JavaScript Code:
/**
* Defines a function `test` to check if a string contains all 26 English alphabet letters.
* @param {string} string - The input string to be tested.
* @returns {boolean|string} - Returns true if the string contains all 26 English alphabet letters,
* otherwise returns a message indicating that the input must be a string.
*/
const test = (string) => {
// Check if the input parameter is a string
if (typeof string !== 'string') {
return 'It must be a string.';
}
// Create a new Set to store unique uppercase characters from the string
const result = new Set();
// Loop through each character in the string, converting it to uppercase
for (const ch of string.toUpperCase()) {
// Check if the character is an uppercase English alphabet letter
if (/[A-Z]/.test(ch)) {
// Add the character to the result set if it is an alphabet letter
result.add(ch);
}
}
// Check if the result set size is equal to 26 (i.e., contains all 26 English alphabet letters)
return result.size === 26;
};
// Test the `test` function with different input strings and log the results
console.log(test("The quick brown fox jumps over the lazy dog"));
console.log(test("Waltz, bad nymph, for quick jigs vex."));
console.log(test("The five boxing wizards jump quickly."));
console.log(test("The boxing wizards jump quickly."));
console.log(test(12356));
Output:
true true true false It must be a string.
Explanation:
In the exercise above,
The function test() first checks if the input parameter 'string' is not a string using the 'typeof' operator. If it's not a string, the function returns a message indicating that it must be a string.
- If the input is a string, the function creates a new "Set" object named 'result'. This set will store unique uppercase characters from the input string.
- The function iterates over each character in the input string (string.toUpperCase()), converting each character to uppercase.
- Within the loop, it checks if the current character is an uppercase English alphabet letter using a regular expression (/[A-Z]/). If the character is an alphabet letter, it adds it to the result set.
- After iterating over all characters in the string, the function checks if the size of the 'result' set is equal to 26. If it is, it means that the input string contains all 26 English alphabet letters, and the function returns 'true'. Otherwise, it returns 'false'.
- The function is then tested with various input strings using console.log.
Flowchart:
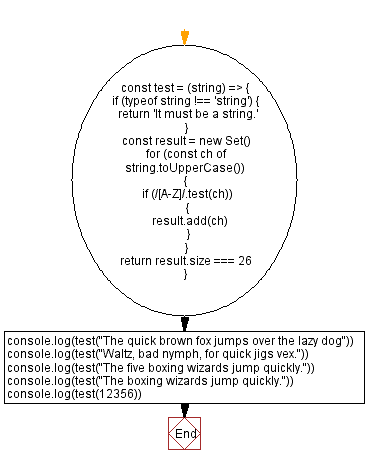
Live Demo:
See the Pen javascript-string-exercise-55 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that checks if a string contains every letter of the alphabet at least once, ignoring case.
- Write a JavaScript function that uses a Set to collect unique letters from a string and compares its size to 26.
- Write a JavaScript function that removes non-alphabet characters before testing for pangram status.
- Write a JavaScript function that validates the input and returns an error if it is not a string.
Improve this sample solution and post your code through Disqus.
Previous: Check a string is in Kebab case.
Next: Check a string is in Pascal case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.